What's New in JavaScript: Exploring Set Methods for Comparing Set-like Objects
JavaScript continues to evolve, with recent updates introducing powerful new functions for the Set object. These methods allow developers to efficiently compare set-like objects, enhancing the functionality of Set for common use cases such as determining intersections, unions, and differences. In this article, we'll explore these new methods, complete with examples and visual aids to demonstrate their utility. Introduction to New Set Methods The following new methods have been added to the Set prototype: Set.prototype.intersection() Set.prototype.union() Set.prototype.difference() Set.prototype.isSubsetOf() Set.prototype.isSupersetOf() Set.prototype.symmetricDifference() These methods simplify complex set operations and make code more readable and maintainable. Set Operations in Action Let’s dive into each of these new methods with examples. 1. Intersection The intersection() method returns a new Set containing elements common to both sets. const setA = new Set([1, 2, 3, 4]); const setB = new Set([3, 4, 5, 6]); const intersectionSet = setA.intersection(setB); console.log(intersectionSet); // Output: Set { 3, 4 } Visualization Set A Set B Intersection 1, 2, 3, 4 3, 4, 5, 6 3, 4 2. Union The union() method combines elements from both sets, removing duplicates. const unionSet = setA.union(setB); console.log(unionSet); // Output: Set { 1, 2, 3, 4, 5, 6 } Visualization Set A Set B Union 1, 2, 3, 4 3, 4, 5, 6 1, 2, 3, 4, 5, 6 3. Difference The difference() method returns a new Set with elements in the first set but not in the second. const differenceSet = setA.difference(setB); console.log(differenceSet); // Output: Set { 1, 2 } Visualization Set A Set B Difference (A - B) 1, 2, 3, 4 3, 4, 5, 6 1, 2 4. Subset and Superset isSubsetOf() Checks if all elements of the set are contained in another set. console.log(new Set([1, 2]).isSubsetOf(setA)); // Output: true isSupersetOf() Checks if the set contains all elements of another set. console.log(setA.isSupersetOf(new Set([1, 2]))); // Output: true 5. Symmetric Difference The symmetricDifference() method returns elements present in either of the sets but not in both. const symmetricDiffSet = setA.symmetricDifference(setB); console.log(symmetricDiffSet); // Output: Set { 1, 2, 5, 6 } Visualization Set A Set B Symmetric Difference 1, 2, 3, 4 3, 4, 5, 6 1, 2, 5, 6 6. Disjoint Check The isDisjointFrom() method checks if two sets have no elements in common. It returns true if the sets are disjoint (i.e., their intersection is empty), and false otherwise. Example: const setA = new Set([1, 2, 3]); const setB = new Set([4, 5, 6]); const setC = new Set([3, 4, 5]); console.log(setA.isDisjointFrom(setB)); // Output: true console.log(setA.isDisjointFrom(setC)); // Output: false Explanation: setA and setB have no overlapping elements, so they are disjoint. setA and setC share the element 3, so they are not disjoint. Visualization Set A Set B Are Disjoint? 1, 2, 3 4, 5, 6 ✅ Yes 1, 2, 3 3, 4, 5 ❌ No Summary of New Methods To recap, here are the new Set methods added to JavaScript and their purposes: intersection(): Finds common elements between two sets. union(): Combines all unique elements from two sets. difference(): Returns elements in the first set but not in the second. symmetricDifference(): Finds elements in either set but not both. isSubsetOf(): Checks if a set is a subset of another set. isSupersetOf(): Checks if a set is a superset of another set. isDisjointFrom(): Checks if two sets have no elements in common. Advantages of These Methods Improved Readability: Simplifies common operations compared to using manual loops or custom logic. Code Efficiency: Optimized implementation for set operations, ensuring better performance. Ease of Use: Unified and intuitive API for comparing and manipulating set-like objects. Real-World Application These methods can be used in various scenarios, such as: Filtering datasets in applications. Identifying common preferences or exclusions in recommendation systems. Comparing permissions between user roles. Conclusion The addition of these new methods to the Set object is a significant enhancement, making JavaScript a more robust language for data manipulation. Whether you're dealing with simple collections or performing complex operations, these methods streamline your workflow and improve the developer experience. What do you think of these updates? Have you used them in your projects? Share your thoughts!

JavaScript continues to evolve, with recent updates introducing powerful new functions for the Set
object. These methods allow developers to efficiently compare set-like objects, enhancing the functionality of Set
for common use cases such as determining intersections, unions, and differences. In this article, we'll explore these new methods, complete with examples and visual aids to demonstrate their utility.
Introduction to New Set Methods
The following new methods have been added to the Set
prototype:
-
Set.prototype.intersection()
-
Set.prototype.union()
-
Set.prototype.difference()
-
Set.prototype.isSubsetOf()
-
Set.prototype.isSupersetOf()
Set.prototype.symmetricDifference()
These methods simplify complex set operations and make code more readable and maintainable.
Set Operations in Action
Let’s dive into each of these new methods with examples.
1. Intersection
The intersection()
method returns a new Set
containing elements common to both sets.
const setA = new Set([1, 2, 3, 4]);
const setB = new Set([3, 4, 5, 6]);
const intersectionSet = setA.intersection(setB);
console.log(intersectionSet); // Output: Set { 3, 4 }
Visualization
Set A | Set B | Intersection |
---|---|---|
1, 2, 3, 4 | 3, 4, 5, 6 | 3, 4 |
2. Union
The union()
method combines elements from both sets, removing duplicates.
const unionSet = setA.union(setB);
console.log(unionSet); // Output: Set { 1, 2, 3, 4, 5, 6 }
Visualization
Set A | Set B | Union |
---|---|---|
1, 2, 3, 4 | 3, 4, 5, 6 | 1, 2, 3, 4, 5, 6 |
3. Difference
The difference()
method returns a new Set
with elements in the first set but not in the second.
const differenceSet = setA.difference(setB);
console.log(differenceSet); // Output: Set { 1, 2 }
Visualization
Set A | Set B | Difference (A - B) |
---|---|---|
1, 2, 3, 4 | 3, 4, 5, 6 | 1, 2 |
4. Subset and Superset
isSubsetOf()
Checks if all elements of the set are contained in another set.
console.log(new Set([1, 2]).isSubsetOf(setA)); // Output: true
isSupersetOf()
Checks if the set contains all elements of another set.
console.log(setA.isSupersetOf(new Set([1, 2]))); // Output: true
5. Symmetric Difference
The symmetricDifference()
method returns elements present in either of the sets but not in both.
const symmetricDiffSet = setA.symmetricDifference(setB);
console.log(symmetricDiffSet); // Output: Set { 1, 2, 5, 6 }
Visualization
Set A | Set B | Symmetric Difference |
---|---|---|
1, 2, 3, 4 | 3, 4, 5, 6 | 1, 2, 5, 6 |
6. Disjoint Check
The isDisjointFrom()
method checks if two sets have no elements in common. It returns true
if the sets are disjoint (i.e., their intersection is empty), and false
otherwise.
Example:
const setA = new Set([1, 2, 3]);
const setB = new Set([4, 5, 6]);
const setC = new Set([3, 4, 5]);
console.log(setA.isDisjointFrom(setB)); // Output: true
console.log(setA.isDisjointFrom(setC)); // Output: false
Explanation:
-
setA
andsetB
have no overlapping elements, so they are disjoint. -
setA
andsetC
share the element3
, so they are not disjoint.
Visualization
Set A | Set B | Are Disjoint? |
---|---|---|
1, 2, 3 | 4, 5, 6 | ✅ Yes |
1, 2, 3 | 3, 4, 5 | ❌ No |
Summary of New Methods
To recap, here are the new Set
methods added to JavaScript and their purposes:
-
intersection()
: Finds common elements between two sets. -
union()
: Combines all unique elements from two sets. -
difference()
: Returns elements in the first set but not in the second. -
symmetricDifference()
: Finds elements in either set but not both. -
isSubsetOf()
: Checks if a set is a subset of another set. -
isSupersetOf()
: Checks if a set is a superset of another set. -
isDisjointFrom()
: Checks if two sets have no elements in common.
Advantages of These Methods
- Improved Readability: Simplifies common operations compared to using manual loops or custom logic.
- Code Efficiency: Optimized implementation for set operations, ensuring better performance.
- Ease of Use: Unified and intuitive API for comparing and manipulating set-like objects.
Real-World Application
These methods can be used in various scenarios, such as:
- Filtering datasets in applications.
- Identifying common preferences or exclusions in recommendation systems.
- Comparing permissions between user roles.
Conclusion
The addition of these new methods to the Set
object is a significant enhancement, making JavaScript a more robust language for data manipulation. Whether you're dealing with simple collections or performing complex operations, these methods streamline your workflow and improve the developer experience.
What do you think of these updates? Have you used them in your projects? Share your thoughts!
What's Your Reaction?
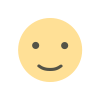
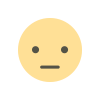
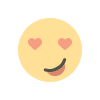
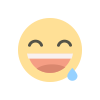
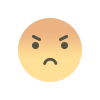
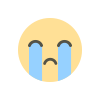
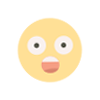