Transactions in Microservices: Part 1 - SAGA Patterns overview.
Distributed systems are powerful yet challenging, especially when it comes to ensuring consistency across multiple services. In a world of microservices, traditional database transactions often fall short. This is where distributed transactions step in. Distributed transactions allow systems to coordinate multiple services while handling failures gracefully. One of the most effective approaches to achieving this is the SAGA pattern, which provides two distinct implementations: Choreography and Orchestration. This article is the first in a series exploring distributed transactions and the SAGA pattern. By the end, you’ll understand its core concepts, when to use it, and how it can be applied in real-world scenarios. A practical example in Go is included to demonstrate the Orchestration approach. The Challenge of Distributed Transactions Imagine building a distributed application where multiple services collaborate to complete a business operation. Here are some examples: Finance: Managing a multi-step loan approval process. E-commerce: Coordinating order placement, payment processing, and shipping. Healthcare: Handling a multi-step workflow for scheduling a medical procedure. Key Challenges Partial Failures: One service may fail while others succeed, leaving the system in an inconsistent state. Data Consistency: The final state must reflect the intended outcome, regardless of individual service failures. Complex Workflows: Coordinating multiple services in a reliable and maintainable way. Traditional database transactions are inadequate for solving these challenges in distributed systems, necessitating patterns like SAGA. The SAGA Pattern: A Reliable Solution The SAGA pattern breaks a complex workflow into smaller, independent steps. Each step performs a specific task and can be compensated (undone) if something goes wrong. Implementation Approaches Choreography: Each service emits events that other services consume to trigger the next step. It is decentralized and event-driven. Orchestration: A central orchestrator coordinates the sequence of steps and manages compensations. It is more centralized and easier to reason about. Both approaches have unique strengths, and the choice depends on the system's requirements. In this article, we’ll focus on Orchestration. Practical Example: Healthcare Workflow with Orchestration Consider a healthcare system managing a multi-step workflow for scheduling a medical procedure. The services involved might include: Patient Management: Verifying patient details and insurance coverage. Appointment Scheduler: Booking an available slot for the procedure. Inventory Management: Reserving medical supplies for the procedure. Billing: Charging the patient or insurer. To ensure consistency across these services, we can use the SAGA pattern with Orchestration. Below is a runnable Go implementation. Code Example: SAGA with Orchestration in Go package main import ( "fmt" "log" ) // Define step and compensation types type Step func() error type Compensation func() // Saga structure type Saga struct { steps []Step compensations []Compensation } func (s *Saga) AddStep(step Step, compensation Compensation) { s.steps = append(s.steps, step) s.compensations = append([]Compensation{compensation}, s.compensations...) } func (s *Saga) Execute() { for i, step := range s.steps { if err := step(); err != nil { log.Printf("Step %d failed: %v. Rolling back...\n", i+1, err) for _, compensation := range s.compensations { compensation() } return } } fmt.Println("Transaction completed successfully!") } func main() { saga := &Saga{} // Step 1: Verify patient and insurance details saga.AddStep( func() error { fmt.Println("Step 1: Verifying patient and insurance details...") // Simulate success return nil }, func() { fmt.Println("Compensation 1: Notify patient of verification failure.") }, ) // Step 2: Schedule procedure saga.AddStep( func() error { fmt.Println("Step 2: Scheduling procedure...") // Simulate success return nil }, func() { fmt.Println("Compensation 2: Cancel procedure schedule.") }, ) // Step 3: Reserve medical supplies saga.AddStep( func() error { fmt.Println("Step 3: Reserving medical supplies...") // Simulate success return nil }, func() { fmt.Println("Compensation 3: Release reserved supplies.") }, ) // Step 4: Process billing saga.AddStep( func() error { fmt.Println("Step 4: Processing billing...") // Simulate failure return fmt.Errorf("billing service unavailable") },

Distributed systems are powerful yet challenging, especially when it comes to ensuring consistency across multiple services. In a world of microservices, traditional database transactions often fall short. This is where distributed transactions step in.
Distributed transactions allow systems to coordinate multiple services while handling failures gracefully. One of the most effective approaches to achieving this is the SAGA pattern, which provides two distinct implementations: Choreography and Orchestration.
This article is the first in a series exploring distributed transactions and the SAGA pattern. By the end, you’ll understand its core concepts, when to use it, and how it can be applied in real-world scenarios. A practical example in Go is included to demonstrate the Orchestration approach.
The Challenge of Distributed Transactions
Imagine building a distributed application where multiple services collaborate to complete a business operation. Here are some examples:
- Finance: Managing a multi-step loan approval process.
- E-commerce: Coordinating order placement, payment processing, and shipping.
- Healthcare: Handling a multi-step workflow for scheduling a medical procedure.
Key Challenges
- Partial Failures: One service may fail while others succeed, leaving the system in an inconsistent state.
- Data Consistency: The final state must reflect the intended outcome, regardless of individual service failures.
- Complex Workflows: Coordinating multiple services in a reliable and maintainable way.
Traditional database transactions are inadequate for solving these challenges in distributed systems, necessitating patterns like SAGA.
The SAGA Pattern: A Reliable Solution
The SAGA pattern breaks a complex workflow into smaller, independent steps. Each step performs a specific task and can be compensated (undone) if something goes wrong.
Implementation Approaches
- Choreography: Each service emits events that other services consume to trigger the next step. It is decentralized and event-driven.
- Orchestration: A central orchestrator coordinates the sequence of steps and manages compensations. It is more centralized and easier to reason about.
Both approaches have unique strengths, and the choice depends on the system's requirements. In this article, we’ll focus on Orchestration.
Practical Example: Healthcare Workflow with Orchestration
Consider a healthcare system managing a multi-step workflow for scheduling a medical procedure. The services involved might include:
- Patient Management: Verifying patient details and insurance coverage.
- Appointment Scheduler: Booking an available slot for the procedure.
- Inventory Management: Reserving medical supplies for the procedure.
- Billing: Charging the patient or insurer.
To ensure consistency across these services, we can use the SAGA pattern with Orchestration. Below is a runnable Go implementation.
Code Example: SAGA with Orchestration in Go
package main
import (
"fmt"
"log"
)
// Define step and compensation types
type Step func() error
type Compensation func()
// Saga structure
type Saga struct {
steps []Step
compensations []Compensation
}
func (s *Saga) AddStep(step Step, compensation Compensation) {
s.steps = append(s.steps, step)
s.compensations = append([]Compensation{compensation}, s.compensations...)
}
func (s *Saga) Execute() {
for i, step := range s.steps {
if err := step(); err != nil {
log.Printf("Step %d failed: %v. Rolling back...\n", i+1, err)
for _, compensation := range s.compensations {
compensation()
}
return
}
}
fmt.Println("Transaction completed successfully!")
}
func main() {
saga := &Saga{}
// Step 1: Verify patient and insurance details
saga.AddStep(
func() error {
fmt.Println("Step 1: Verifying patient and insurance details...")
// Simulate success
return nil
},
func() { fmt.Println("Compensation 1: Notify patient of verification failure.") },
)
// Step 2: Schedule procedure
saga.AddStep(
func() error {
fmt.Println("Step 2: Scheduling procedure...")
// Simulate success
return nil
},
func() { fmt.Println("Compensation 2: Cancel procedure schedule.") },
)
// Step 3: Reserve medical supplies
saga.AddStep(
func() error {
fmt.Println("Step 3: Reserving medical supplies...")
// Simulate success
return nil
},
func() { fmt.Println("Compensation 3: Release reserved supplies.") },
)
// Step 4: Process billing
saga.AddStep(
func() error {
fmt.Println("Step 4: Processing billing...")
// Simulate failure
return fmt.Errorf("billing service unavailable")
},
func() { fmt.Println("Compensation 4: Reverse any pending charges.") },
)
// Execute the saga
saga.Execute()
}
The example provided above is a simplified implementation, primarily intended to demonstrate the basic workings of a SAGA with orchestration. While it is not suitable for direct use in real-world scenarios, it should give you a solid understanding of the core concepts.
In the upcoming articles of this series, we will explore more advanced, real-world examples to deepen your understanding.
Stay tuned!
Applications Beyond Healthcare
The SAGA pattern is versatile and widely applicable:
- Finance: Automating loan approvals across multiple systems.
- Logistics: Managing shipment tracking and order fulfillment.
- E-commerce: Handling complex order workflows involving payment, inventory, and delivery.
Key Takeaways
- Distributed transactions are vital for maintaining consistency in microservices.
- The SAGA pattern simplifies the coordination of these transactions.
- Choreography and Orchestration each offer unique advantages depending on system requirements.
In the next article, we’ll explore the Choreography approach, diving into its event-driven nature with another practical example in Go.
Check out the full repository for runnable examples.
Have questions or feedback? Let’s discuss in the comments!
What's Your Reaction?
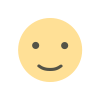
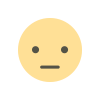
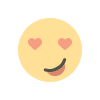
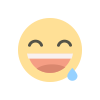
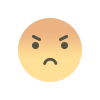
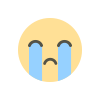
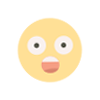