Could any professional object-oriented programmer review this code snippet?
The following code snippet is written in Java. Could anyone review it? @Test public void testAccountInsert() { LOG.info("Starting testAccountImport run"); List importRows = new ArrayList(); // insert new account with null status info -- should auto-create open date LOG.info("1: import new account with null status info -- should auto-create open date"); insertRows.add(new HashMap(Map.of(P_SHORT_NAME, SHORT_NAME, P_ACCOUNTS, getAccString(ACC_NEW_222, null, null, null) ))); getServices().getGeneralImporter().process(importRows); commitTransaction(); List accounts = services.getPortfolioService().loadPortfolio(ID).getAccounts(); Account account = accounts.stream().filter(a -> a.getNumber().equals(ACC_NEW_111)).findFirst().orElse(new Account()); assertEquals(Account.AccountStatus.OPEN, account.getAccountStatus()); assertEquals(TODAY, account.getOpenDate()); importRows.clear(); // insert new account with empty status -- should auto-create open date LOG.info("2: import new account with empty status -- should auto-create open date"); insertRows.add(new HashMap(Map.of(P_SHORT_NAME, SHORT_NAME, getAccString(ACC_NEW_333, EMPTY, null, null)))); getServices().getGeneralImporter().process(importRows); commitTransaction(); List accounts1 = services.getPortfolioService().loadPortfolio(ID).getAccounts(); Account account1 = accounts1.stream().filter(a -> a.getNumber().equals(ACC_NEW_444)).findFirst().orElse(new Account()); assertEquals(Account.AccountStatus.OPEN, account1.getAccountStatus()); assertEquals(TODAY, account1.getOpenDate()); insertRows.clear(); // update existing closed account -- import with empty status value -- no update LOG.info("3: update existing closed account -- import with empty status value -- no update"); insertRows.add(new HashMap(Map.of(P_SHORT_NAME, SHORT_NAME, getAcc(ACC_DEFAULT_CLOSED, EMPTY, null, null)))); getServices().getGeneralImporter().process(importRows); commitTransaction(); List accounts2 = services.getPortfolioService().loadPortfolio(ID).getAccounts(); Account accountClosed = accounts2.stream().filter(a -> a.getNumber().equals(ACC_DEFAULT_CLOSED)).findFirst().orElse(new Account()); assertEquals(Account.AccountStatus.CLOSED, accountClosed.getAccountStatus()); assertEquals(DEFAULT_ACC_CLOSED_OPENDATE, sdf.format(accountClosed.getOpenDate())); assertEquals(DEFAULT_ACC_CLOSED_CLOSEDATE, sdf.format(accountClosed.getCloseDate())); insertRows.clear(); //... } Note that this test method is only half of the original method, i.e. there are still more than a hundred lines of code the the end of this single test method
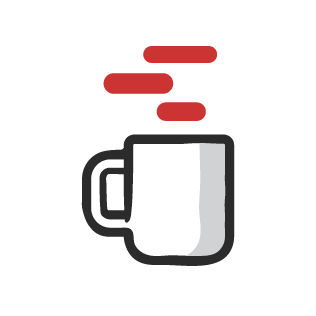
The following code snippet is written in Java. Could anyone review it?
@Test
public void testAccountInsert() {
LOG.info("Starting testAccountImport run");
List
Note that this test method is only half of the original method, i.e. there are still more than a hundred lines of code the the end of this single test method
What's Your Reaction?
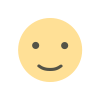
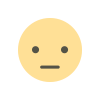
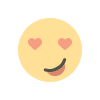
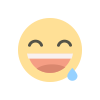
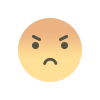
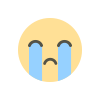
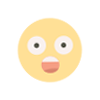