Securing Your Next.js Application: The Basic Defenders (Security Headers)
Original Post The Essential Security Headers Every Next.js Application Needs As seasoned developers, we know that building a robust and feature-rich application is only half the battle. The other, equally critical half, is ensuring its security. While the complex world of cybersecurity can feel overwhelming, especially for those just starting, there are fundamental steps we can take to significantly harden our applications against common attacks. One such powerful and relatively straightforward technique is the implementation of HTTP security headers. Think of security headers as the initial line of defense for your Next.js application. They act like instructions to the user's browser, dictating how it should behave when interacting with your website. By carefully configuring these headers, we can proactively mitigate a range of vulnerabilities, including Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and the risk of clickjacking through iframe embedding. This article will focus on the essential security headers every Next.js application should implement, regardless of its size or complexity. We'll break down each header, explain its purpose, why it's crucial, and how to implement it in your Next.js project. Importantly, remember that this is a foundational layer. Securing your application is an ongoing journey, and this is just the first, but vital, step. Why Bother with Security Headers? (The Real-World Impact) Before diving into the specifics, let's address the "why." Why should you, as a developer, spend time configuring these seemingly obscure headers? The answer lies in the real-world impact of common web vulnerabilities: Cross-Site Scripting (XSS): Imagine a malicious actor injecting harmful JavaScript code into your website. This code could then be executed in the browsers of your unsuspecting users, allowing attackers to steal login credentials, cookies, or even redirect users to malicious sites. Resource: OWASP on XSS. The consequences can range from defacing your website to severe data breaches and reputational damage. Cross-Site Request Forgery (CSRF): In a CSRF attack, a malicious website, email, blog, instant message, or program tricks a user's web browser into performing an unwanted action on a trusted site when the user is authenticated. For example, changing their password or making a purchase without their knowledge. Resource: OWASP on CSRF. This can lead to unauthorized actions and financial losses for your users. Clickjacking (via iframe Embedding): Attackers can embed your website within an iframe on a malicious page. They can then overlay invisible elements on top of your content, tricking users into clicking on actions they didn't intend. Think of it like an invisible button placed over a legitimate "Confirm Purchase" button. Resource: OWASP on Clickjacking. This can be used for various malicious purposes, including stealing credentials or performing unwanted actions. By implementing the right security headers, you're essentially providing the browser with instructions to defend against these attacks. It's like equipping your application with a basic security detail. The Essential Security Headers for Your Next.js App: Let's now explore the core security headers you should be implementing: 1. Content Security Policy (CSP): Your Shield Against XSS The Content Security Policy (CSP) is arguably the most powerful security header for preventing XSS attacks. It works by defining a whitelist of trusted sources from which the browser is allowed to load resources (scripts, styles, images, etc.). If the browser encounters a resource from an untrusted source, it will block it. Why it's necessary: XSS attacks exploit the browser's trust in code that originates from your domain. CSP explicitly tells the browser what sources are legitimate, reducing the attack surface significantly. How it works: You define CSP directives that specify allowed sources for different resource types. For example: Content-Security-Policy: default-src 'self'; script-src 'self' 'unsafe-inline' https://example.com; style-src 'self' https://fonts.googleapis.com; img-src 'self' data:; default-src 'self';: By default, only load resources from the same origin as the document. script-src 'self' 'unsafe-inline' https://example.com;: Allow scripts from the same origin, inline scripts (use with caution!), and scripts from https://example.com. style-src 'self' https://fonts.googleapis.com;: Allow styles from the same origin and https://fonts.googleapis.com. img-src 'self' data:;: Allow images from the same origin and data URIs (for inline images). Important Considerations: CSP is not a one-size-fits-all solution. You need to carefully tailor your CSP based on the specific resources your application uses. Be strict but not too strict. An overly restrictive CSP can break your website. Start with a reporting-only policy (using Content-Security-

The Essential Security Headers Every Next.js Application Needs
As seasoned developers, we know that building a robust and feature-rich application is only half the battle. The other, equally critical half, is ensuring its security. While the complex world of cybersecurity can feel overwhelming, especially for those just starting, there are fundamental steps we can take to significantly harden our applications against common attacks. One such powerful and relatively straightforward technique is the implementation of HTTP security headers.
Think of security headers as the initial line of defense for your Next.js application. They act like instructions to the user's browser, dictating how it should behave when interacting with your website. By carefully configuring these headers, we can proactively mitigate a range of vulnerabilities, including Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and the risk of clickjacking through iframe embedding.
This article will focus on the essential security headers every Next.js application should implement, regardless of its size or complexity. We'll break down each header, explain its purpose, why it's crucial, and how to implement it in your Next.js project. Importantly, remember that this is a foundational layer. Securing your application is an ongoing journey, and this is just the first, but vital, step.
Why Bother with Security Headers? (The Real-World Impact)
Before diving into the specifics, let's address the "why." Why should you, as a developer, spend time configuring these seemingly obscure headers? The answer lies in the real-world impact of common web vulnerabilities:
Cross-Site Scripting (XSS): Imagine a malicious actor injecting harmful JavaScript code into your website. This code could then be executed in the browsers of your unsuspecting users, allowing attackers to steal login credentials, cookies, or even redirect users to malicious sites. Resource: OWASP on XSS. The consequences can range from defacing your website to severe data breaches and reputational damage.
Cross-Site Request Forgery (CSRF): In a CSRF attack, a malicious website, email, blog, instant message, or program tricks a user's web browser into performing an unwanted action on a trusted site when the user is authenticated. For example, changing their password or making a purchase without their knowledge. Resource: OWASP on CSRF. This can lead to unauthorized actions and financial losses for your users.
Clickjacking (via iframe Embedding): Attackers can embed your website within an iframe on a malicious page. They can then overlay invisible elements on top of your content, tricking users into clicking on actions they didn't intend. Think of it like an invisible button placed over a legitimate "Confirm Purchase" button. Resource: OWASP on Clickjacking. This can be used for various malicious purposes, including stealing credentials or performing unwanted actions.
By implementing the right security headers, you're essentially providing the browser with instructions to defend against these attacks. It's like equipping your application with a basic security detail.
The Essential Security Headers for Your Next.js App:
Let's now explore the core security headers you should be implementing:
1. Content Security Policy (CSP): Your Shield Against XSS
The Content Security Policy (CSP) is arguably the most powerful security header for preventing XSS attacks. It works by defining a whitelist of trusted sources from which the browser is allowed to load resources (scripts, styles, images, etc.). If the browser encounters a resource from an untrusted source, it will block it.
Why it's necessary: XSS attacks exploit the browser's trust in code that originates from your domain. CSP explicitly tells the browser what sources are legitimate, reducing the attack surface significantly.
How it works: You define CSP directives that specify allowed sources for different resource types. For example:
Content-Security-Policy: default-src 'self'; script-src 'self' 'unsafe-inline' https://example.com; style-src 'self' https://fonts.googleapis.com; img-src 'self' data:;
-
default-src 'self';
: By default, only load resources from the same origin as the document. -
script-src 'self' 'unsafe-inline' https://example.com;
: Allow scripts from the same origin, inline scripts (use with caution!), and scripts fromhttps://example.com
. -
style-src 'self' https://fonts.googleapis.com;
: Allow styles from the same origin andhttps://fonts.googleapis.com
. -
img-src 'self' data:;
: Allow images from the same origin and data URIs (for inline images).
Important Considerations:
- CSP is not a one-size-fits-all solution. You need to carefully tailor your CSP based on the specific resources your application uses.
- Be strict but not too strict. An overly restrictive CSP can break your website. Start with a reporting-only policy (using
Content-Security-Policy-Report-Only
) to identify potential issues before enforcing it. - Inline scripts and styles should be avoided whenever possible. They weaken the effectiveness of CSP.
- Nonce and Hash-based CSP: For more fine-grained control over inline scripts and styles, consider using nonces or hashes. Resource: Google on CSP Nonces.
2. Strict-Transport-Security (HSTS): Enforcing HTTPS
The Strict-Transport-Security (HSTS) header forces browsers to interact with your website exclusively over HTTPS. This prevents man-in-the-middle attacks where attackers might try to downgrade the connection to HTTP to eavesdrop on traffic.
Why it's necessary: HTTPS provides encryption and authentication, protecting user data in transit. HSTS ensures that even if a user accidentally types http://
or clicks an old HTTP link, their browser will automatically upgrade the connection to HTTPS.
How it works:
Strict-Transport-Security: max-age=31536000; includeSubDomains; preload
-
max-age=31536000
: Tells the browser to remember this rule for one year (value is in seconds). -
includeSubDomains
: Applies the HSTS policy to all subdomains. -
preload
: Allows you to submit your domain to the HSTS preload list, which is built into browsers. This ensures HSTS is enforced even on the very first visit. Resource: HSTS Preload List.
Important Considerations:
- Ensure your website is fully accessible via HTTPS before enabling HSTS.
- Start with a shorter
max-age
value and gradually increase it. - Consider the
preload
directive for enhanced security.
3. X-Frame-Options: Preventing Clickjacking
The X-Frame-Options header tells the browser whether or not it should allow your website to be framed within an ,
, or
. This is crucial for preventing clickjacking attacks.
Why it's necessary: By controlling iframe embedding, you prevent malicious websites from embedding your site and tricking users into unintended actions.
How it works:
X-Frame-Options: DENY
or
X-Frame-Options: SAMEORIGIN
or
X-Frame-Options: ALLOW-FROM https://trusted-domain.com
-
DENY
: Prevents your page from being framed by any site. This is the safest option for most applications. -
SAMEORIGIN
: Allows framing only by pages within the same origin as your website. -
ALLOW-FROM uri
: Allows framing only by the specified URI. Note: This directive is deprecated in modern browsers and Content-Security-Policy'sframe-ancestors
directive is the recommended alternative.
Important Considerations:
-
DENY
is generally the recommended setting. Only useSAMEORIGIN
orALLOW-FROM
if you have a legitimate need for framing your content (e.g., embedding in a corporate portal). - Consider using the
frame-ancestors
directive within your CSP for more granular control and wider browser support.
4. X-Content-Type-Options: Preventing MIME Sniffing
The X-Content-Type-Options header instructs the browser to strictly adhere to the Content-Type
header provided by the server. This helps prevent MIME sniffing attacks, where browsers try to guess the content type of a resource, potentially leading to security vulnerabilities if a malicious file is interpreted as executable code.
Why it's necessary: Attackers might try to upload files with misleading extensions or content types. By disabling MIME sniffing, you ensure that the browser treats the file as the server intended.
How it works:
X-Content-Type-Options: nosniff
Important Considerations:
- This header is generally safe to implement and highly recommended.
5. Referrer-Policy: Controlling Referrer Information
The Referrer-Policy header controls how much referrer information (the URL of the previous page) the browser includes when following links from your website. This can help protect user privacy and prevent information leakage.
Why it's necessary: The referrer header can sometimes leak sensitive information about your users' browsing history.
How it works:
Referrer-Policy: strict-origin-when-cross-origin
This is a good default value that sends the origin (scheme, host, and port) in cross-origin requests but only sends the full URL for same-origin requests. Other options include:
-
no-referrer
: Never send the referrer header. -
no-referrer-when-downgrade
: Send the referrer header when navigating from HTTPS to HTTPS, but not from HTTPS to HTTP. -
origin-only
: Send only the origin in the referrer header.
Resource: MDN on Referrer-Policy.
Important Considerations:
- Choose a policy that balances security and functionality. Consider the needs of analytics tools and other services that might rely on referrer information.
Implementing Security Headers in Next.js
There are several ways to implement these security headers in your Next.js application:
-
Next.js Middleware: This is the recommended approach as it allows you to set headers for all routes consistently. You can create a
middleware.ts
file in yoursrc
directory (or at the root if you don't use thesrc
directory) and set the headers there:
```typescript jsx
import { NextResponse } from 'next/server';
import type { NextRequest } from 'next/server';
export function middleware(request: NextRequest) {
const response = NextResponse.next();
response.headers.set(
'Content-Security-Policy',
"default-src 'self'; script-src 'self' 'unsafe-inline' https://example.com; style-src 'self' https://fonts.googleapis.com; img-src 'self' data:;"
);
response.headers.set('Strict-Transport-Security', 'max-age=31536000; includeSubDomains; preload');
response.headers.set('X-Frame-Options', 'DENY');
response.headers.set('X-Content-Type-Options', 'nosniff');
response.headers.set('Referrer-Policy', 'strict-origin-when-cross-origin');
return response;
}
export const config = {
matcher: [
/*
* Match all request paths except for the ones starting with:
* - _next/static (static files)
* - _next/image (image optimization files)
* - favicon.ico (favicon file)
/
'/((?!_next/static|_next/image|favicon.ico).)',
],
};
* **`next.config.js` (Less Recommended for Dynamic Headers):** You can also set headers in your `next.config.js` file, but this is less flexible for dynamic CSP configurations.
```javascript
/** @type {import('next').NextConfig} */
const nextConfig = {
async headers() {
return [
{
source: '/:path*',
headers: [
{
key: 'Content-Security-Policy',
value: "default-src 'self'; script-src 'self' 'unsafe-inline' https://example.com; style-src 'self' https://fonts.googleapis.com; img-src 'self' data:;",
},
{
key: 'Strict-Transport-Security',
value: 'max-age=31536000; includeSubDomains; preload',
},
{
key: 'X-Frame-Options',
value: 'DENY',
},
{
key: 'X-Content-Type-Options',
value: 'nosniff',
},
{
key: 'Referrer-Policy',
value: 'strict-origin-when-cross-origin',
},
],
},
];
},
};
module.exports = nextConfig;
- Reverse Proxy (e.g., Nginx, Apache): If you're using a reverse proxy in front of your Next.js application, you can configure security headers at the proxy level. This can be useful for managing headers across multiple applications.
Beyond the Basics: Your Security Journey Continues
Implementing these basic security headers is a significant step towards securing your Next.js application. However, it's crucial to understand that this is not the end of the road. Depending on the sensitivity of your data and the complexity of your application, you'll likely need to explore further security measures:
- CSRF Tokens: Implement anti-CSRF tokens for state-changing requests.
- Input Validation and Output Encoding: Sanitize user input to prevent injection attacks.
- Regular Security Audits: Conduct periodic security assessments to identify potential vulnerabilities.
- Dependency Management: Keep your dependencies up to date to patch known security flaws.
- Rate Limiting and Authentication/Authorization: Implement measures to prevent brute-force attacks and control access to your application.
Conclusion: A Foundation for a Secure Future
Securing your Next.js application is an ongoing process, not a one-time task. By implementing these fundamental security headers, you're laying a solid foundation for a more secure and resilient application. While the initial configuration might seem daunting, the benefits in terms of preventing common attacks and protecting your users are well worth the effort.
Remember to tailor your header configurations to your specific needs, test your implementation thoroughly, and continuously learn about evolving security threats and best practices. This proactive approach will empower you to build safer and more trustworthy web applications. Start with these basic defenders, and continue your journey towards a more secure future for your Next.js projects.
What's Your Reaction?
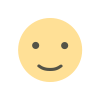
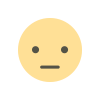
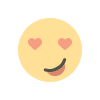
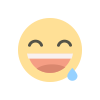
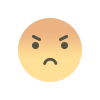
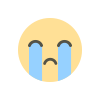
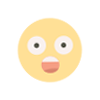