DOM in JavaScript
DOCUMENT OBJECT MODEL (DOM) The DOM API is a programming interface for web documents. It represents the page so that programs can change the document structure, style and content. The DOM represents the document as a tree of objects; each object represents a part of the page. DOM Manipulations in JavaScript These are some of the basics we can consider while implementing DOM in JavaScript; 1.Query selector; This is a greedy selector.It selects the first element which has been specified. Forexample; querySelector("h1") This will target the first h1 element throughout the whole html document 2.QueryselectorAll: This targets all h3 elements in the html document. Forexample; querySelectorAll("h3") These will be output in a node list in the console. NB: Node list looks like an array but the two are significantly different A nodelist is a browser API yet an array is a JavaScript API. In short, node list does not belong to JavaScript, for that case , loops can not be implemented on it. Also, getElementbyTagName() and QueryselectorAll are not JavaScript methods but rather browser APIs. INNER HTML AND INNER TEXT It is advisable to use inner html unlike inner text because, in such a case, modifications of your script by other people would be limited. Ways of targetting elements We have many ways of targeting or selecting elements we want to manipulate in our document. Some of these ways include: By Id By Name By Class By Tag By Tag name NB: We never use Id and Class to select anything from the browser. We therefore use the Queryselector. Key Concepts in JavaScript. a) HOISTING:Elaborating this using an example below, Forexample: 1.Console.log(a); 2.var a; 3.a = 5; 4.Console log a; During execution in the console, a is going to be output as undefined for line number 1. This means that even though we didn't explicitly declare a initially, it was "magically" declared in the browser , and this is called hoisting. Normally, for line number 4, the output would be 5 since a was explicitly initialized. b) CLOSURE:This is created every time a function is created. c) GLOBAL EXECUTION CONTEXT:The Global Execution Context is the environment where your JavaScript code runs when it is not inside any function. d) CALL STACK:When a script calls a function, the interpreter adds it to the stack and then starts carrying out the function My Github link Linked in

DOCUMENT OBJECT MODEL (DOM)
The DOM API is a programming interface for web documents. It represents the page so that programs can change the document structure, style and content.
The DOM represents the document as a tree of objects; each object represents a part of the page.
DOM Manipulations in JavaScript
These are some of the basics we can consider while implementing DOM in JavaScript;
1.Query selector; This is a greedy selector.It selects the first element which has been specified.
Forexample;
querySelector("h1")
This will target the first h1 element throughout the whole html document
2.QueryselectorAll: This targets all h3 elements in the html document.
Forexample;
querySelectorAll("h3")
These will be output in a node list in the console.
NB: Node list looks like an array but the two are significantly different
A nodelist is a browser API yet an array is a JavaScript API.
In short, node list does not belong to JavaScript, for that case , loops can not be implemented on it.
Also, getElementbyTagName() and QueryselectorAll are not JavaScript methods but rather browser APIs.
INNER HTML AND INNER TEXT
It is advisable to use inner html unlike inner text because, in such a case, modifications of your script by other people would be limited.
Ways of targetting elements
- We have many ways of targeting or selecting elements we want to manipulate in our document.
Some of these ways include:
By Id
By Name
By Class
By Tag
By Tag name
NB: We never use Id and Class to select anything from the browser.
We therefore use the Queryselector.
Key Concepts in JavaScript.
a) HOISTING:Elaborating this using an example below,
Forexample:
1.Console.log(a);
2.var a;
3.a = 5;
4.Console log a;
During execution in the console, a is going to be output as undefined for line number 1.
This means that even though we didn't explicitly declare a
initially, it was "magically" declared in the browser , and this is called hoisting.
Normally, for line number 4, the output would be 5 since
a
was explicitly initialized.
b) CLOSURE:This is created every time a function is created.
c) GLOBAL EXECUTION CONTEXT:The Global Execution Context is the environment where your JavaScript code runs when it is not inside any function.
d) CALL STACK:When a script calls a function, the interpreter adds it to the stack and then starts carrying out the function
What's Your Reaction?
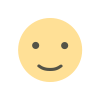
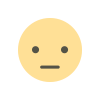
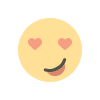
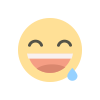
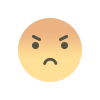
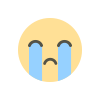
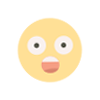