Scope of var, let, const
What is Scope? Scope of variable refers to the region of the program where the variable is accessible. Understanding variable scope is crucial for writing efficient and error-free code. In JavaScript, there are three types of variable scope: var let const var The var keyword was first introduced in 1995 with initial release of JavaScript. The value initiated with var keyword has a global scope, which means the value is accessible by all parts of the file. var x = 10 function inner() { console.log(x) // output: 10 } console.log(x) // output: 10 Variable declared using var is hoisted to top the file which means you can try to access the variable before it initialized, but as an output it will give undefined because we didn't initialize the value to the variable. console.log(x) // output: undefined var x = 10 console.log(x) // output: 10 let The let keyword was introduced in 2015, where the keyword is added to ECMAScript 6 to give block scope functionality. The value initialized using let give block scope. So, the variable can't be accessed in any other scope. example: function, global scope. Unlike var, variable declared using let keyword when the value accessed before declaration raises an error because of temporal dead zone. console.log(x) // output: raises error let x = 10 Also, you can't be able to access block level variable inside the function too. let x = 10 function inner() { console.log(x) // output: raises error } const This is also another type of block scope variable, in addition to that variable declared with const should be initialized on the same line. const PI = 3.14 console.log(3.14) // output: 3.14 This keyword is introduced in ECMAScript 6, this is used to store constant value line environmental variables, access tokens, etc. Mainly used to store any constant value won't change in future. If you tried to change the value of const declared variable, then it will raise TypeError: Assignment to constant variable in runtime. const PI = 3.14 PI = 3.5 // output: TypeError: Assignment to constant variable. Happy Coding...

What is Scope?
Scope of variable refers to the region of the program where the variable is accessible. Understanding variable scope is crucial for writing efficient and error-free code.
In JavaScript, there are three types of variable scope:
- var
- let
- const
var
The
var
keyword was first introduced in 1995 with initial release of JavaScript.The value initiated with var keyword has a global scope, which means the value is accessible by all parts of the file.
var x = 10
function inner() {
console.log(x) // output: 10
}
console.log(x) // output: 10
- Variable declared using
var
is hoisted to top the file which means you can try to access the variable before it initialized, but as an output it will giveundefined
because we didn't initialize the value to the variable.
console.log(x) // output: undefined
var x = 10
console.log(x) // output: 10
let
The
let
keyword was introduced in 2015, where the keyword is added to ECMAScript 6 to give block scope functionality.The value initialized using
let
give block scope. So, the variable can't be accessed in any other scope. example: function, global scope.Unlike
var
, variable declared usinglet
keyword when the value accessed before declaration raises an error because of temporal dead zone.
console.log(x) // output: raises error
let x = 10
- Also, you can't be able to access block level variable inside the function too.
let x = 10
function inner() {
console.log(x) // output: raises error
}
const
- This is also another type of block scope variable, in addition to that variable declared with
const
should be initialized on the same line.
const PI = 3.14
console.log(3.14) // output: 3.14
This keyword is introduced in ECMAScript 6, this is used to store constant value line environmental variables, access tokens, etc. Mainly used to store any constant value won't change in future.
If you tried to change the value of
const
declared variable, then it will raiseTypeError: Assignment to constant variable
in runtime.
const PI = 3.14
PI = 3.5 // output: TypeError: Assignment to constant variable.
Happy Coding...
What's Your Reaction?
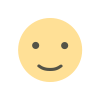
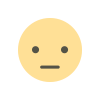
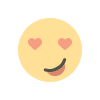
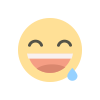
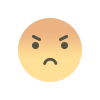
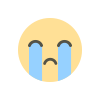
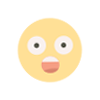