Solving `Uncaught (in promise) ReferenceError: exports is not defined` in Nuxt.js with Vee-Validate
If you're working on a Nuxt.js project and encounter the error: Uncaught (in promise) ReferenceError: exports is not defined ...when using vee-validate, you're not alone! This issue commonly occurs due to improper transpilation of the vee-validate library, which uses modern ES modules. Here's how to fix it and ensure everything works smoothly. Why This Happens The error occurs because Nuxt.js (especially when running in SSR mode) sometimes needs explicit instructions to transpile modern libraries like vee-validate. Without this, the library may try to use exports, a CommonJS feature, in an ES module environment, resulting in the error. The Solution 1. Ensure Compatible Versions Before jumping to configurations, confirm you're using the right versions for your Nuxt and Vue setup: Nuxt 3 + Vue 3: Use vee-validate v4. Nuxt 2 + Vue 2: Use vee-validate v3, unless you're using the Vue Composition API plugin, which can work with v4. 2. Transpile Vee-Validate Add vee-validate to the transpile option in your nuxt.config. For Nuxt 3: // nuxt.config.ts export default defineNuxtConfig({ build: { transpile: ['vee-validate'] } }) For Nuxt 2: // nuxt.config.js export default { build: { transpile: ['vee-validate'] } } This ensures that Nuxt will properly transform vee-validate for both client and server environments. 3. Example Form Setup with Vee-Validate Here’s a quick example of using vee-validate with yup for schema validation in Nuxt 3: import { useForm, useField } from 'vee-validate' import * as yup from 'yup' // Define the validation schema const formSchema = yup.object({ email: yup.string().required('Email is required').email('Invalid email'), password: yup.string().required('Password is required').min(6, 'Password must be at least 6 characters') }) // Initialize the form with the schema const { handleSubmit, errors } = useForm({ validationSchema: formSchema }) // Define form fields const { value: email, errorMessage: emailError } = useField('email') const { value: password, errorMessage: passwordError } = useField('password') // Handle form submission const onSubmit = handleSubmit((values) => { console.log('Form values:', values) }) Email {{ emailError }} Password {{ passwordError }} Submit 4. Check Your Node Version Ensure you're running Node.js 14 or later, as older versions might not handle modern ES module syntax well. Final Thoughts By adding vee-validate to the transpile configuration, you can avoid the frustrating exports is not defined error and fully leverage the power of vee-validate in your Nuxt.js project. If you're still experiencing issues, double-check your package versions and project setup. Happy coding!

If you're working on a Nuxt.js project and encounter the error:
Uncaught (in promise) ReferenceError: exports is not defined
...when using vee-validate
, you're not alone! This issue commonly occurs due to improper transpilation of the vee-validate
library, which uses modern ES modules. Here's how to fix it and ensure everything works smoothly.
Why This Happens
The error occurs because Nuxt.js (especially when running in SSR mode) sometimes needs explicit instructions to transpile modern libraries like vee-validate
. Without this, the library may try to use exports
, a CommonJS feature, in an ES module environment, resulting in the error.
The Solution
1. Ensure Compatible Versions
Before jumping to configurations, confirm you're using the right versions for your Nuxt and Vue setup:
- Nuxt 3 + Vue 3: Use vee-validate v4.
- Nuxt 2 + Vue 2: Use vee-validate v3, unless you're using the Vue Composition API plugin, which can work with v4.
2. Transpile Vee-Validate
Add vee-validate
to the transpile
option in your nuxt.config
.
For Nuxt 3:
// nuxt.config.ts
export default defineNuxtConfig({
build: {
transpile: ['vee-validate']
}
})
For Nuxt 2:
// nuxt.config.js
export default {
build: {
transpile: ['vee-validate']
}
}
This ensures that Nuxt will properly transform vee-validate
for both client and server environments.
3. Example Form Setup with Vee-Validate
Here’s a quick example of using vee-validate
with yup
for schema validation in Nuxt 3:
<script setup lang="ts">
import { useForm, useField } from 'vee-validate'
import * as yup from 'yup'
// Define the validation schema
const formSchema = yup.object({
email: yup.string().required('Email is required').email('Invalid email'),
password: yup.string().required('Password is required').min(6, 'Password must be at least 6 characters')
})
// Initialize the form with the schema
const { handleSubmit, errors } = useForm({
validationSchema: formSchema
})
// Define form fields
const { value: email, errorMessage: emailError } = useField('email')
const { value: password, errorMessage: passwordError } = useField('password')
// Handle form submission
const onSubmit = handleSubmit((values) => {
console.log('Form values:', values)
})
script>
<template>
template>
4. Check Your Node Version
Ensure you're running Node.js 14 or later, as older versions might not handle modern ES module syntax well.
Final Thoughts
By adding vee-validate
to the transpile configuration, you can avoid the frustrating exports is not defined
error and fully leverage the power of vee-validate in your Nuxt.js project. If you're still experiencing issues, double-check your package versions and project setup.
Happy coding!
What's Your Reaction?
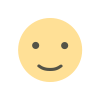
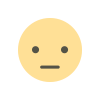
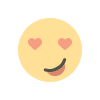
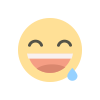
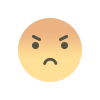
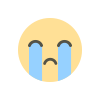
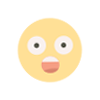