useMemo in ReactJs
At the very starting after listening useMemo what come in mind at first is, what is useMemo? So, useMemo is the hook in react that helps on memoizating the computed value or the return result of the function. It is used for the optimization of the react component. Ok optimization, let's discuss how useMemo is helping in optimization. As we know react rerenders the component after the change in state. Because of which many unnecessary function or other operations that are heavy which are not linked to the state may execute too. import { useState } from "react"; const App = () => { const [count, setCount] = useState(0); const [item, setItem] = useState(1); let changingCount = count + 1; console.log("UpdatedCount:", changingCount); return ( State: {count} Item: {item} setItem(item + 1)}>Increase Item setCount(count + 1)}>Increase Count ); }; export default App; As we can see in the snippet above, my application is being rerender after I am clicking the button. Let's focus on the point console.log in application. My console is executing every time I am clicking any button. Wait, but my console is depending on my state "count". What can I do so that I can execute my console only when my "count" state is updating. Yeah, exactly we can use useMemo hook for that. Let's use it now. import { useMemo, useState } from "react"; const App = () => { const [count, setCount] = useState(0); const [item, setItem] = useState(1); useMemo(() => { let changingCount = count + 1; console.log("UpdatedCount:", changingCount); }, [count]); return ( State: {count} Item: {item} setItem(item + 1)}>Increase Item setCount(count + 1)}>Increase Count ); }; export default App; What benefit did we get by using useMemo hook. So, as you can see here useMemo has two dependencies first one is a callback function and the second one is the dependency array. Now my function or the console is only executing when my count state is being updated which means the function is now dependent on the state. Wow, is my application being optimized? Yes of course we tackle the unnecessary computation of our application. Callback function and dependency array as parameter. Heard it write? Yeah it sounds similar to the useEffect but understand both have their own usage where useEffect is used for handling sideEffects in reacts. Wait wait, what is sideEffect? SideEffect is the interaction of the application with the external substance like api fetching and using events. Where useMemo is used to tackle the unnecessary computations.

At the very starting after listening useMemo what come in mind at first is, what is useMemo? So, useMemo is the hook in react that helps on memoizating the computed value or the return result of the function. It is used for the optimization of the react component. Ok optimization, let's discuss how useMemo is helping in optimization. As we know react rerenders the component after the change in state. Because of which many unnecessary function or other operations that are heavy which are not linked to the state may execute too.
import { useState } from "react";
const App = () => {
const [count, setCount] = useState(0);
const [item, setItem] = useState(1);
let changingCount = count + 1;
console.log("UpdatedCount:", changingCount);
return (
<>
State: {count}
Item: {item}
>
);
};
export default App;
As we can see in the snippet above, my application is being rerender after I am clicking the button. Let's focus on the point console.log in application. My console is executing every time I am clicking any button. Wait, but my console is depending on my state "count". What can I do so that I can execute my console only when my "count" state is updating. Yeah, exactly we can use useMemo hook for that. Let's use it now.
import { useMemo, useState } from "react";
const App = () => {
const [count, setCount] = useState(0);
const [item, setItem] = useState(1);
useMemo(() => {
let changingCount = count + 1;
console.log("UpdatedCount:", changingCount);
}, [count]);
return (
<>
State: {count}
Item: {item}
>
);
};
export default App;
What benefit did we get by using useMemo hook. So, as you can see here useMemo has two dependencies first one is a callback function and the second one is the dependency array. Now my function or the console is only executing when my count state is being updated which means the function is now dependent on the state. Wow, is my application being optimized? Yes of course we tackle the unnecessary computation of our application.
Callback function and dependency array as parameter. Heard it write? Yeah it sounds similar to the useEffect but understand both have their own usage where useEffect is used for handling sideEffects in reacts. Wait wait, what is sideEffect? SideEffect is the interaction of the application with the external substance like api fetching and using events. Where useMemo is used to tackle the unnecessary computations.
What's Your Reaction?
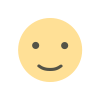
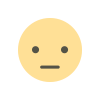
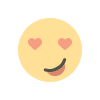
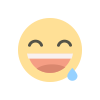
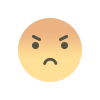
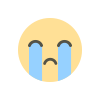
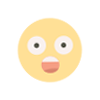