Overview of Backend Architectures (Monolith vs Microservices)
Overview of Backend Architectures: Monolith vs. Microservices Introduction: Choosing the right backend architecture is crucial for application scalability and maintainability. Two dominant approaches are monolithic and microservices architectures. This article provides a brief overview of each. Monolithic Architecture: A monolithic architecture comprises all application components (database, user interface, business logic) as a single unit. Deployment involves deploying the entire application as one package. Prerequisites: Strong understanding of a single programming language and framework. Relatively smaller team size. Simple application functionality. Advantages: Easier to develop and deploy initially. Simpler debugging and testing. Better data consistency due to centralized database. Disadvantages: Difficult to scale individual components. Scaling requires scaling the entire application. Technology stack lock-in. Changing a single component requires redeploying the entire application. Slow development cycles due to complex dependencies. Microservices Architecture: Microservices decompose an application into small, independent services, each focused on a specific business function. These services communicate via APIs (e.g., REST). Prerequisites: Expertise in multiple programming languages and frameworks. Larger, specialized development teams. Complex application with distinct functionalities. Advantages: Independent scaling of individual services. Technology diversity; different services can use different technologies. Faster development cycles due to independent deployments. Enhanced fault isolation; failure of one service doesn't bring down the entire system. Disadvantages: Increased complexity in development, deployment, and monitoring. Data consistency challenges across multiple services. Requires robust API gateways and service discovery mechanisms. Features (Illustrative Example): A simple user authentication microservice might look like this (Python with Flask): from flask import Flask, request, jsonify app = Flask(__name__) # ... authentication logic ... @app.route('/authenticate', methods=['POST']) def authenticate(): # ... authentication code ... return jsonify({'token': 'your_token'}) if __name__ == '__main__': app.run(debug=True) Conclusion: The choice between monolithic and microservices architecture depends on the application's complexity, team size, and scalability requirements. Monoliths are suitable for smaller, simpler applications, while microservices are better suited for large, complex applications that require high scalability and flexibility. Careful consideration of these factors is crucial for long-term success.

Overview of Backend Architectures: Monolith vs. Microservices
Introduction:
Choosing the right backend architecture is crucial for application scalability and maintainability. Two dominant approaches are monolithic and microservices architectures. This article provides a brief overview of each.
Monolithic Architecture:
A monolithic architecture comprises all application components (database, user interface, business logic) as a single unit. Deployment involves deploying the entire application as one package.
Prerequisites:
- Strong understanding of a single programming language and framework.
- Relatively smaller team size.
- Simple application functionality.
Advantages:
- Easier to develop and deploy initially.
- Simpler debugging and testing.
- Better data consistency due to centralized database.
Disadvantages:
- Difficult to scale individual components. Scaling requires scaling the entire application.
- Technology stack lock-in. Changing a single component requires redeploying the entire application.
- Slow development cycles due to complex dependencies.
Microservices Architecture:
Microservices decompose an application into small, independent services, each focused on a specific business function. These services communicate via APIs (e.g., REST).
Prerequisites:
- Expertise in multiple programming languages and frameworks.
- Larger, specialized development teams.
- Complex application with distinct functionalities.
Advantages:
- Independent scaling of individual services.
- Technology diversity; different services can use different technologies.
- Faster development cycles due to independent deployments.
- Enhanced fault isolation; failure of one service doesn't bring down the entire system.
Disadvantages:
- Increased complexity in development, deployment, and monitoring.
- Data consistency challenges across multiple services.
- Requires robust API gateways and service discovery mechanisms.
Features (Illustrative Example):
A simple user authentication microservice might look like this (Python with Flask):
from flask import Flask, request, jsonify
app = Flask(__name__)
# ... authentication logic ...
@app.route('/authenticate', methods=['POST'])
def authenticate():
# ... authentication code ...
return jsonify({'token': 'your_token'})
if __name__ == '__main__':
app.run(debug=True)
Conclusion:
The choice between monolithic and microservices architecture depends on the application's complexity, team size, and scalability requirements. Monoliths are suitable for smaller, simpler applications, while microservices are better suited for large, complex applications that require high scalability and flexibility. Careful consideration of these factors is crucial for long-term success.
What's Your Reaction?
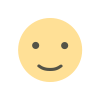
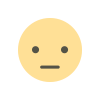
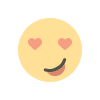
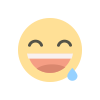
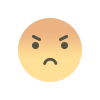
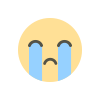
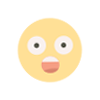