JSON Unescape: Understanding and Using It Effectively
JSON (JavaScript Object Notation) has become the universal standard for data exchange, powering APIs, web services, and more. However, handling escaped characters in JSON strings can be tricky, especially when working with large datasets or complex APIs. JSON unescape simplifies this process by decoding escaped characters, making data more readable and usable. What is JSON Unescape?\ JSON unescape refers to the process of converting escaped characters in a JSON string back into their original, human-readable form. These escaped characters are encoded to ensure that special characters, such as quotation marks or newlines, don’t interfere with JSON syntax. For instance, the string \"Hello\nWorld\" contains escape sequences (\" and \n) that represent double quotes and a newline, respectively. JSON unescape translates these sequences back to their intended representation. Why is JSON Unescape Important?\ When working with JSON data, escaped characters can hinder readability and usability. For example, raw API responses or log files may contain numerous escape sequences that obscure the actual content. Unescaping these characters ensures the data is clean, making it easier to debug, analyze, and process. Furthermore, unescaping is vital for proper data rendering. A failure to decode JSON data accurately can result in display issues or unexpected errors, especially in front-end applications. Common Escaped Characters in JSON\ To better understand JSON unescape, let’s look at some of the most common escaped characters in JSON: Line Breaks (\n): Represents a new line in a string. Tabs (\t): Represents a tab space. Backslashes (\\): Escapes the backslash character itself. Double Quotes (\"): Used to include quotes inside JSON strings. Unicode Characters (\uXXXX): Encodes special characters or symbols in hexadecimal format (e.g., \u00A9 for the © symbol). How JSON Unescape Works\ The unescaping process involves parsing the JSON string and converting the escape sequences into their original forms. For example, a JSON string containing \"Hello\\nWorld\" would be unescaped to: arduino CopyEdit "Hello World" This decoding ensures that data appears in its intended format, whether for display, processing, or storage. Tools and Libraries for JSON Unescape\ A variety of tools and programming libraries are available to help you unescape JSON data effortlessly. These include: Online Tools: Platforms like JSONLint and FreeFormatter allow users to paste JSON data and unescape it with a click. JavaScript: The JSON.parse() method automatically handles unescaping while parsing JSON strings. Python: The json module and functions like html.unescape() can decode escaped JSON data. Java: Libraries like org.json provide built-in methods for parsing and unescaping JSON. JSON Unescape in Popular Programming Languages\ Here’s how JSON unescape can be implemented in some of the most widely used programming languages: JavaScript\ JavaScript’s JSON.parse() automatically unescapes characters: javascript CopyEdit const jsonString = '{"message": "Hello\\nWorld"}'; const parsed = JSON.parse(jsonString); console.log(parsed.message); // Output: Hello // World Python\ Python’s json library makes unescaping seamless: python CopyEdit import json json_string = '{"message": "Hello\\nWorld"}' data = json.loads(json_string) print(data['message']) # Output: Hello # World Java\ Java’s org.json library can decode JSON data effectively: java CopyEdit import org.json.JSONObject; String jsonString = "{\"message\": \"Hello\\nWorld\"}"; JSONObject jsonObject = new JSONObject(jsonString); System.out.println(jsonObject.getString("message")); Best Practices for JSON Unescape\ To ensure efficient handling of escaped characters, follow these best practices: Validate JSON Input: Always validate your JSON data for proper formatting before unescaping. Use Built-In Libraries: Whenever possible, use standard libraries and methods for unescaping to avoid manual errors. Handle Edge Cases: Prepare for scenarios where escape sequences may be incomplete or invalid. Integrate with Workflows: Automate JSON validation and unescaping in your development pipeline to save time. Challenges in JSON Unescaping\ While JSON unescape is a straightforward process, it comes with certain challenges: Invalid JSON Format: Malformed JSON data can lead to parsing errors. Always validate your JSON before attempting unescaping. Encoding Conflicts: Character encoding mismatches (e.g., UTF-8 vs. ASCII) can cause issues during unescaping. Performance Bottlenecks: Working with large datasets containing extensive escape sequences can slow down processing. These challenges can be mitigated by using reliable tools and adhering to best practices. When to Use JSON Unescape\ JSON unescape is particularly useful in scenarios such as: Rendering User Data: Decoding API responses for front-end applications. Debugging L

JSON (JavaScript Object Notation) has become the universal standard for data exchange, powering APIs, web services, and more. However, handling escaped characters in JSON strings can be tricky, especially when working with large datasets or complex APIs. JSON unescape simplifies this process by decoding escaped characters, making data more readable and usable.
What is JSON Unescape?\
JSON unescape refers to the process of converting escaped characters in a JSON string back into their original, human-readable form. These escaped characters are encoded to ensure that special characters, such as quotation marks or newlines, don’t interfere with JSON syntax. For instance, the string \"Hello\nWorld\" contains escape sequences (\" and \n) that represent double quotes and a newline, respectively. JSON unescape translates these sequences back to their intended representation.
Why is JSON Unescape Important?\
When working with JSON data, escaped characters can hinder readability and usability. For example, raw API responses or log files may contain numerous escape sequences that obscure the actual content. Unescaping these characters ensures the data is clean, making it easier to debug, analyze, and process.
Furthermore, unescaping is vital for proper data rendering. A failure to decode JSON data accurately can result in display issues or unexpected errors, especially in front-end applications.
Common Escaped Characters in JSON\
To better understand JSON unescape, let’s look at some of the most common escaped characters in JSON:
- Line Breaks (\n): Represents a new line in a string.
- Tabs (\t): Represents a tab space.
- Backslashes (\\): Escapes the backslash character itself.
- Double Quotes (\"): Used to include quotes inside JSON strings.
- Unicode Characters (\uXXXX): Encodes special characters or symbols in hexadecimal format (e.g., \u00A9 for the © symbol).
How JSON Unescape Works\
The unescaping process involves parsing the JSON string and converting the escape sequences into their original forms. For example, a JSON string containing \"Hello\\nWorld\" would be unescaped to:
arduino
CopyEdit
"Hello
World"
This decoding ensures that data appears in its intended format, whether for display, processing, or storage.
Tools and Libraries for JSON Unescape\
A variety of tools and programming libraries are available to help you unescape JSON data effortlessly. These include:
- Online Tools: Platforms like JSONLint and FreeFormatter allow users to paste JSON data and unescape it with a click.
- JavaScript: The JSON.parse() method automatically handles unescaping while parsing JSON strings.
- Python: The json module and functions like html.unescape() can decode escaped JSON data.
- Java: Libraries like org.json provide built-in methods for parsing and unescaping JSON.
JSON Unescape in Popular Programming Languages\
Here’s how JSON unescape can be implemented in some of the most widely used programming languages:
- JavaScript\ JavaScript’s JSON.parse() automatically unescapes characters:
javascript
CopyEdit
const jsonString = '{"message": "Hello\\nWorld"}';
const parsed = JSON.parse(jsonString);
console.log(parsed.message); // Output: Hello
// World
- Python\ Python’s json library makes unescaping seamless:
python
CopyEdit
import json
json_string = '{"message": "Hello\\nWorld"}'
data = json.loads(json_string)
print(data['message']) # Output: Hello
# World
- Java\ Java’s org.json library can decode JSON data effectively:
java
CopyEdit
import org.json.JSONObject;
String jsonString = "{\"message\": \"Hello\\nWorld\"}";
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject.getString("message"));
Best Practices for JSON Unescape\
To ensure efficient handling of escaped characters, follow these best practices:
- Validate JSON Input: Always validate your JSON data for proper formatting before unescaping.
- Use Built-In Libraries: Whenever possible, use standard libraries and methods for unescaping to avoid manual errors.
- Handle Edge Cases: Prepare for scenarios where escape sequences may be incomplete or invalid.
- Integrate with Workflows: Automate JSON validation and unescaping in your development pipeline to save time.
Challenges in JSON Unescaping\
While JSON unescape is a straightforward process, it comes with certain challenges:
- Invalid JSON Format: Malformed JSON data can lead to parsing errors. Always validate your JSON before attempting unescaping.
- Encoding Conflicts: Character encoding mismatches (e.g., UTF-8 vs. ASCII) can cause issues during unescaping.
- Performance Bottlenecks: Working with large datasets containing extensive escape sequences can slow down processing.
These challenges can be mitigated by using reliable tools and adhering to best practices.
When to Use JSON Unescape\
JSON unescape is particularly useful in scenarios such as:
- Rendering User Data: Decoding API responses for front-end applications.
- Debugging Logs: Making JSON log files more human-readable.
- Data Transformation: Preparing JSON data for migration or further processing.
Conclusion\
JSON unescape is a crucial tool for developers working with JSON data, ensuring clean and readable outputs. Whether you’re debugging, processing API responses, or transforming data, understanding how to unescape JSON will save time and prevent errors. By leveraging built-in libraries, tools, and best practices, you can seamlessly handle escaped characters and enhance your workflows.
What's Your Reaction?
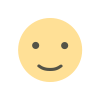
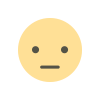
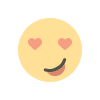
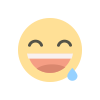
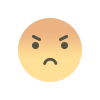
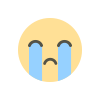
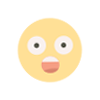