JSON-Server for Next.js 15: Everything You Need to Know
In this guide, we'll explore how to use JSON Server to create a mock API and integrate it into a Next.js 15 application with TypeScript. We'll cover the setup process, explain each HTTP method (GET, POST, PUT, DELETE), and provide clear examples for each, alongside the new use feature in Next.js. What is JSON Server? JSON Server allows you to quickly create a RESTful API using a simple JSON file. It supports: CRUD Operations: GET, POST, PUT, DELETE. Dynamic Routes: Customize endpoints for complex scenarios. Ease of Use: Start with minimal setup. Why Use JSON Server with Next.js 15? Frontend-First Development: Build UI components before the backend is ready. Rapid Prototyping: Test features without waiting for backend integration. Custom API Behavior: Simulate backend behavior with ease. Setting Up JSON Server 1. Install JSON Server In your Next.js project, install JSON Server: npm install --save-dev json-server This installs JSON Server as a development dependency. 2. Create the Database File Create a db.json file in your project root: { "users": [ { "id": 1, "name": "John Doe", "email": "john@example.com" }, { "id": 2, "name": "Jane Smith", "email": "jane@example.com" } ] } 3. Configure JSON Server Add a script to package.json to run the server: "scripts": { "json-server": "json-server --watch db.json --port 4000" } Using --delay The --delay flag allows you to simulate network latency for API calls. For example: "scripts": { "json-server": "json-server --watch db.json --port 4000 --delay 1000" } This adds a 1-second delay (1000 milliseconds) to all API responses, helping you test loading states in your frontend application. Run the server with: npm run json-server Your API is now live at http://localhost:4000. Working with HTTP Methods 1. GET: Fetching Data The GET method retrieves data. Here's how to fetch users: 'use client'; import { use } from 'react'; async function fetchUsers() { const res = await fetch('http://localhost:4000/users'); if (!res.ok) { throw new Error('Failed to fetch users'); } return res.json(); } export default function UsersPage() { const users = use(fetchUsers()); return ( Users {users.map((user: { id: number; name: string }) => ( {user.name} ))} ); } Explanation: fetchUsers: Fetches data from the mock API. use: A React hook introduced in Next.js 15 to handle server-side data fetching. 2. POST: Adding Data The POST method adds new records. Example: 'use client'; import { useState } from 'react'; export default function AddUser() { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const handleAddUser = async () => { const res = await fetch('http://localhost:4000/users', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name, email }), }); if (res.ok) { alert('User added successfully!'); } }; return ( Add New User setName(e.target.value)} /> setEmail(e.target.value)} /> Add User ); } 3. PUT: Updating Data The PUT method updates an existing record: 'use client'; import { useState } from 'react'; export default function UpdateUser() { const [id, setId] = useState(''); const [name, setName] = useState(''); const [email, setEmail] = useState(''); const handleUpdateUser = async () => { const res = await fetch(`http://localhost:4000/users/${id}`, { method: 'PUT', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name, email }), }); if (res.ok) { alert('User updated successfully!'); } }; return ( Update User setId(e.target.value)} /> setName(e.target.value)} /> setEmail(e.target.value)} /> Update User ); } 4. DELETE: Deleting Data The DELETE method removes a record: 'use client'; import { useState } from 'react'; export default function DeleteUser() { const [id, setId] = useState(''); const handleDeleteUser = async () => { const res = await fetch(`http://localhost:4000/users/${id}`, { method: 'DELETE', }); if (res.ok) { alert('User deleted successfully!'); } }; return ( Delete User setId(e.target.value)} /> Delete User ); } Best Practices Port Management: Use a custom port to avoid conflicts with Next.js (default: 3000). Error Handling: Implement proper error handling in all API calls. TypeScript Types: Define types for data to ensure type safety. Environment Variables: Store API URLs in .env files for flexibility. Simulate Latency: Use --delay to test loading st

In this guide, we'll explore how to use JSON Server to create a mock API and integrate it into a Next.js 15 application with TypeScript. We'll cover the setup process, explain each HTTP method (GET
, POST
, PUT
, DELETE
), and provide clear examples for each, alongside the new use
feature in Next.js.
What is JSON Server?
JSON Server allows you to quickly create a RESTful API using a simple JSON file. It supports:
-
CRUD Operations:
GET
,POST
,PUT
,DELETE
. - Dynamic Routes: Customize endpoints for complex scenarios.
- Ease of Use: Start with minimal setup.
Why Use JSON Server with Next.js 15?
- Frontend-First Development: Build UI components before the backend is ready.
- Rapid Prototyping: Test features without waiting for backend integration.
- Custom API Behavior: Simulate backend behavior with ease.
Setting Up JSON Server
1. Install JSON Server
In your Next.js project, install JSON Server:
npm install --save-dev json-server
This installs JSON Server as a development dependency.
2. Create the Database File
Create a db.json
file in your project root:
{
"users": [
{ "id": 1, "name": "John Doe", "email": "john@example.com" },
{ "id": 2, "name": "Jane Smith", "email": "jane@example.com" }
]
}
3. Configure JSON Server
Add a script to package.json
to run the server:
"scripts": {
"json-server": "json-server --watch db.json --port 4000"
}
Using --delay
The --delay
flag allows you to simulate network latency for API calls. For example:
"scripts": {
"json-server": "json-server --watch db.json --port 4000 --delay 1000"
}
This adds a 1-second delay (1000 milliseconds) to all API responses, helping you test loading states in your frontend application.
Run the server with:
npm run json-server
Your API is now live at http://localhost:4000
.
Working with HTTP Methods
1. GET
: Fetching Data
The GET
method retrieves data. Here's how to fetch users:
'use client';
import { use } from 'react';
async function fetchUsers() {
const res = await fetch('http://localhost:4000/users');
if (!res.ok) {
throw new Error('Failed to fetch users');
}
return res.json();
}
export default function UsersPage() {
const users = use(fetchUsers());
return (
<div>
<h1>Usersh1>
<ul>
{users.map((user: { id: number; name: string }) => (
<li key={user.id}>{user.name}li>
))}
ul>
div>
);
}
Explanation:
-
fetchUsers
: Fetches data from the mock API. -
use
: A React hook introduced in Next.js 15 to handle server-side data fetching.
2. POST
: Adding Data
The POST
method adds new records. Example:
'use client';
import { useState } from 'react';
export default function AddUser() {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleAddUser = async () => {
const res = await fetch('http://localhost:4000/users', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ name, email }),
});
if (res.ok) {
alert('User added successfully!');
}
};
return (
<div>
<h2>Add New Userh2>
<input
type="text"
placeholder="Name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<input
type="email"
placeholder="Email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<button onClick={handleAddUser}>Add Userbutton>
div>
);
}
3. PUT
: Updating Data
The PUT
method updates an existing record:
'use client';
import { useState } from 'react';
export default function UpdateUser() {
const [id, setId] = useState('');
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const handleUpdateUser = async () => {
const res = await fetch(`http://localhost:4000/users/${id}`, {
method: 'PUT',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ name, email }),
});
if (res.ok) {
alert('User updated successfully!');
}
};
return (
<div>
<h2>Update Userh2>
<input
type="text"
placeholder="ID"
value={id}
onChange={(e) => setId(e.target.value)}
/>
<input
type="text"
placeholder="Name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<input
type="email"
placeholder="Email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<button onClick={handleUpdateUser}>Update Userbutton>
div>
);
}
4. DELETE
: Deleting Data
The DELETE
method removes a record:
'use client';
import { useState } from 'react';
export default function DeleteUser() {
const [id, setId] = useState('');
const handleDeleteUser = async () => {
const res = await fetch(`http://localhost:4000/users/${id}`, {
method: 'DELETE',
});
if (res.ok) {
alert('User deleted successfully!');
}
};
return (
<div>
<h2>Delete Userh2>
<input
type="text"
placeholder="ID"
value={id}
onChange={(e) => setId(e.target.value)}
/>
<button onClick={handleDeleteUser}>Delete Userbutton>
div>
);
}
Best Practices
- Port Management: Use a custom port to avoid conflicts with Next.js (default: 3000).
- Error Handling: Implement proper error handling in all API calls.
- TypeScript Types: Define types for data to ensure type safety.
-
Environment Variables: Store API URLs in
.env
files for flexibility. -
Simulate Latency: Use
--delay
to test loading states and simulate slower networks.
Conclusion
JSON Server is a powerful tool for frontend developers working with Next.js 15. It provides an easy way to simulate APIs and accelerate development workflows. With examples for all HTTP methods, you now have everything needed to integrate JSON Server into your Next.js projects effectively.
What's Your Reaction?
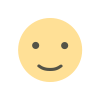
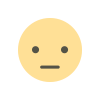
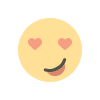
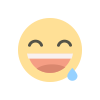
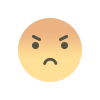
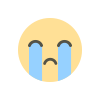
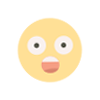