How Programming Will Look In the Future?
Programming hasn't fundamentally changed since the 1940s. Most languages still follow the von Neumann paradigm: write sequential instructions and modify data in memory. Let's look at a simple example: total = 0 for number in range(1, 6): total += number print(total) # Outputs: 15 Simple and familiar, right? But there's a problem. Modern computers aren't getting faster like they used to. Instead, they're getting more cores, and our traditional programming model isn't great at handling multiple cores efficiently. The Multi-Core Challenge We've tried to adapt with tools for concurrent programming. Take Go's goroutines: package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup numbers := []int{1, 2, 3, 4, 5} for _, n := range numbers { wg.Add(1) go func(x int) { defer wg.Done() fmt.Printf("Processing %d\n", x) }(n) } wg.Wait() fmt.Println("All done!") } These solutions help, but they introduce problems like race conditions, deadlocks, and complex state management. We're building on top of a paradigm that wasn't designed for parallel execution, and control-flow languages are inherently hard to visualize. A Different Approach: Data Flow Programming What if we could write programs differently? Imagine describing your program as a network of independent nodes that pass data to each other - no shared state, just data flowing through a system: Everything runs in parallel by default Data is immutable, preventing race conditions Program structure mirrors data flow Natural scaling across multiple cores Enter Nevalang Nevalang does exactly that. It's a modern language built around dataflow that treats programs as message-passing graphs rather than sequences of instructions: import { fmt } def Main(start any) (stop any) { for_print For{Print} wait Wait --- :start -> 1..5 -> for_print -> wait -> :stop } Here we define a component Main that receives a start signal and sends a stop signal. It has two nodes: for_print (a For with Print inside) and wait. When started, it creates a stream of numbers 1-5, prints each through for_print, waits for completion, and sends the stop signal. Visualized: Nevalang represents a fundamental shift in programming, embracing a paradigm naturally suited for parallelism and visualization rather than fighting traditional programming limitations. Try Nevalang on GitHub Make just a little bit shorter, but not too muchThe future of programming might not look like the past. As our hardware continues to evolve, our programming models need to evolve too. Nevalang shows us one exciting possibility for that future. WARNING: Nevalang is currently in development and not yet production-ready. We're looking for contributors and early-adopters. Join! This is an ambitious project maintained by a small group of enthusiasts. Your support by joining us will show interest and motivate us to continue. Discord Reddit Telegram Twitter Please give us a star ⭐️ to increase our chances of getting into GitHub trends - the more attention Nevalang gets, the higher our chances of actually making a difference.

Programming hasn't fundamentally changed since the 1940s. Most languages still follow the von Neumann paradigm: write sequential instructions and modify data in memory. Let's look at a simple example:
total = 0
for number in range(1, 6):
total += number
print(total) # Outputs: 15
Simple and familiar, right? But there's a problem. Modern computers aren't getting faster like they used to. Instead, they're getting more cores, and our traditional programming model isn't great at handling multiple cores efficiently.
The Multi-Core Challenge
We've tried to adapt with tools for concurrent programming. Take Go's goroutines:
package main
import (
"fmt"
"sync"
)
func main() {
var wg sync.WaitGroup
numbers := []int{1, 2, 3, 4, 5}
for _, n := range numbers {
wg.Add(1)
go func(x int) {
defer wg.Done()
fmt.Printf("Processing %d\n", x)
}(n)
}
wg.Wait()
fmt.Println("All done!")
}
These solutions help, but they introduce problems like race conditions, deadlocks, and complex state management. We're building on top of a paradigm that wasn't designed for parallel execution, and control-flow languages are inherently hard to visualize.
A Different Approach: Data Flow Programming
What if we could write programs differently? Imagine describing your program as a network of independent nodes that pass data to each other - no shared state, just data flowing through a system:
- Everything runs in parallel by default
- Data is immutable, preventing race conditions
- Program structure mirrors data flow
- Natural scaling across multiple cores
Enter Nevalang
Nevalang does exactly that. It's a modern language built around dataflow that treats programs as message-passing graphs rather than sequences of instructions:
import { fmt }
def Main(start any) (stop any) {
for_print For{Print}
wait Wait
---
:start -> 1..5 -> for_print -> wait -> :stop
}
Here we define a component Main
that receives a start
signal and sends a stop
signal. It has two nodes: for_print
(a For
with Print
inside) and wait
. When started, it creates a stream of numbers 1-5, prints each through for_print
, waits for completion, and sends the stop
signal.
Visualized:
Nevalang represents a fundamental shift in programming, embracing a paradigm naturally suited for parallelism and visualization rather than fighting traditional programming limitations.
Make just a little bit shorter, but not too muchThe future of programming might not look like the past. As our hardware continues to evolve, our programming models need to evolve too. Nevalang shows us one exciting possibility for that future.
WARNING: Nevalang is currently in development and not yet production-ready. We're looking for contributors and early-adopters. Join!
This is an ambitious project maintained by a small group of enthusiasts. Your support by joining us will show interest and motivate us to continue.
Please give us a star ⭐️ to increase our chances of getting into GitHub trends - the more attention Nevalang gets, the higher our chances of actually making a difference.
What's Your Reaction?
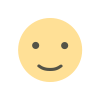
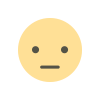
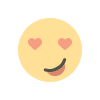
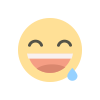
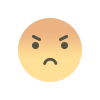
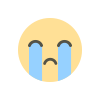
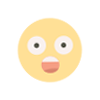