DSA Journey with Python - Day 1 (Python Array 01)
Okay, let's dive into arrays in Python! I'll break it down for you, keeping it beginner-friendly with simple examples and a touch of visual thinking to help solidify your understanding. What is an Array? Think of an array as a container for storing a sequence of items of the same type. Imagine a row of numbered boxes where each box holds a single item. Key features: Ordered: Items have a specific position (index) starting from 0. Same type: Typically, arrays hold elements of the same data type (e.g., all numbers, all strings). Fixed-size (in some languages): Once you create an array, its size is fixed in many languages. Python lists are dynamically sized, which makes them a more flexible option. Fast access: You can quickly access any item using its index. Python's "Array" (Lists) In Python, we use lists to represent arrays. Lists are more flexible than traditional arrays, because they are dynamically sized (can grow or shrink). Example 1: Creating and Accessing a List # Creating a list of numbers numbers = [10, 20, 30, 40, 50] # Visual Representation: # [ 10, 20, 30, 40, 50 ] # ^ ^ ^ ^ ^ # 0 1 2 3 4 (Indexes) # Accessing elements by index first_number = numbers[0] # Accesses the element at index 0 (value 10) third_number = numbers[2] # Accesses the element at index 2 (value 30) print(f"First number: {first_number}") print(f"Third number: {third_number}") # Negative Indexing last_number = numbers[-1] # Accesses the last element, another way of accessing numbers[4] second_last_number = numbers[-2] # Accesses the second last element, another way of accessing numbers[3] print(f"Last number: {last_number}") print(f"Second Last number: {second_last_number}") Visual: Imagine the numbers list as boxes in a row: +---+---+---+---+---+ | 10| 20| 30| 40| 50| +---+---+---+---+---+ ^ ^ ^ ^ ^ 0 1 2 3 4 -5 -4 -3 -2 -1 numbers[0] accesses the box at index 0, which contains 10. numbers[2] accesses the box at index 2, which contains 30. Example 2: List with different types of data data = ["apple", 10, True, 23.34] print(data) print(data[0]) print(data[1]) print(data[2]) print(data[3]) # Visual Representation: # [ "apple", 10, True, 23.34] # ^ ^ ^ ^ # 0 1 2 3 Example 3: Modifying List Elements colors = ["red", "green", "blue"] # Visual: # ["red", "green", "blue"] # 0 1 2 colors[1] = "yellow" # Changes the element at index 1 # Visual After Modification: # ["red", "yellow", "blue"] # 0 1 2 print(colors) # Output: ['red', 'yellow', 'blue'] Example 4: Adding and Removing Elements fruits = ["apple", "banana"] # Visual: # ["apple", "banana"] # 0 1 fruits.append("orange") # Adds to the end of the list # Visual After Append: # ["apple", "banana", "orange"] # 0 1 2 fruits.insert(1, "mango") # Adds element at index 1 # Visual after insert: # ["apple", "mango", "banana", "orange"] # 0 1 2 3 print(fruits) removed_fruit = fruits.pop(2) # Removes element at index 2 # Visual after pop: # ["apple", "mango", "orange"] # 0 1 2 print(f"removed element: {removed_fruit}") print(fruits) fruits.remove("orange") # Removes the first element with that value print(fruits) #Visual after remove: #["apple","mango"] # 0 1 Important List Operations len(list): Returns the number of elements in the list. list.append(item): Adds an item to the end. list.insert(index, item): Inserts an item at a specific index. list.pop(index): Removes and returns the item at a specific index (or last item if no index is given). list.remove(item): Removes the first instance of the item. list.clear(): Removes all the items in the list list.reverse(): Reverses the elements in the list item in list: Checks if an item is present in the list (returns True or False). Example 5: Iterating through a List animals = ["dog", "cat", "bird"] # Using a for loop with index for i in range(len(animals)): print(f"Index {i}: {animals[i]}") print("\n") # New line # Using a for loop more simply for animal in animals: print(f"Animal: {animal}") Visualizing the For Loops When using a for loop, it iterates over every element in the list: for i in range(len(animals)): The loop goes through indexes: 0, 1, and 2 animals[0] -> "dog" animals[1] -> "cat" animals[2] -> "bird" for animal in animals: The loop goes through elements directly animal -> "dog" animal -> "cat" animal -> "bird" Let's Recap Arrays (represented by lists in Python) store collections of items. Each item has an index starting from 0. Lists in Python are flexible; you can easily modify them. You can access elements using their index (e.g., my_list[2]). Use methods like append(), insert(), pop(), and remove() to modify list c

Okay, let's dive into arrays in Python! I'll break it down for you, keeping it beginner-friendly with simple examples and a touch of visual thinking to help solidify your understanding.
What is an Array?
Think of an array as a container for storing a sequence of items of the same type. Imagine a row of numbered boxes where each box holds a single item.
Key features:
- Ordered: Items have a specific position (index) starting from 0.
- Same type: Typically, arrays hold elements of the same data type (e.g., all numbers, all strings).
- Fixed-size (in some languages): Once you create an array, its size is fixed in many languages. Python lists are dynamically sized, which makes them a more flexible option.
- Fast access: You can quickly access any item using its index.
Python's "Array" (Lists)
In Python, we use lists to represent arrays. Lists are more flexible than traditional arrays, because they are dynamically sized (can grow or shrink).
Example 1: Creating and Accessing a List
# Creating a list of numbers
numbers = [10, 20, 30, 40, 50]
# Visual Representation:
# [ 10, 20, 30, 40, 50 ]
# ^ ^ ^ ^ ^
# 0 1 2 3 4 (Indexes)
# Accessing elements by index
first_number = numbers[0] # Accesses the element at index 0 (value 10)
third_number = numbers[2] # Accesses the element at index 2 (value 30)
print(f"First number: {first_number}")
print(f"Third number: {third_number}")
# Negative Indexing
last_number = numbers[-1] # Accesses the last element, another way of accessing numbers[4]
second_last_number = numbers[-2] # Accesses the second last element, another way of accessing numbers[3]
print(f"Last number: {last_number}")
print(f"Second Last number: {second_last_number}")
Visual:
Imagine the numbers list as boxes in a row:
+---+---+---+---+---+
| 10| 20| 30| 40| 50|
+---+---+---+---+---+
^ ^ ^ ^ ^
0 1 2 3 4
-5 -4 -3 -2 -1
numbers[0] accesses the box at index 0, which contains 10.
numbers[2] accesses the box at index 2, which contains 30.
Example 2: List with different types of data
data = ["apple", 10, True, 23.34]
print(data)
print(data[0])
print(data[1])
print(data[2])
print(data[3])
# Visual Representation:
# [ "apple", 10, True, 23.34]
# ^ ^ ^ ^
# 0 1 2 3
Example 3: Modifying List Elements
colors = ["red", "green", "blue"]
# Visual:
# ["red", "green", "blue"]
# 0 1 2
colors[1] = "yellow" # Changes the element at index 1
# Visual After Modification:
# ["red", "yellow", "blue"]
# 0 1 2
print(colors) # Output: ['red', 'yellow', 'blue']
Example 4: Adding and Removing Elements
fruits = ["apple", "banana"]
# Visual:
# ["apple", "banana"]
# 0 1
fruits.append("orange") # Adds to the end of the list
# Visual After Append:
# ["apple", "banana", "orange"]
# 0 1 2
fruits.insert(1, "mango") # Adds element at index 1
# Visual after insert:
# ["apple", "mango", "banana", "orange"]
# 0 1 2 3
print(fruits)
removed_fruit = fruits.pop(2) # Removes element at index 2
# Visual after pop:
# ["apple", "mango", "orange"]
# 0 1 2
print(f"removed element: {removed_fruit}")
print(fruits)
fruits.remove("orange") # Removes the first element with that value
print(fruits)
#Visual after remove:
#["apple","mango"]
# 0 1
Important List Operations
- len(list): Returns the number of elements in the list.
- list.append(item): Adds an item to the end.
- list.insert(index, item): Inserts an item at a specific index.
- list.pop(index): Removes and returns the item at a specific index (or last item if no index is given).
- list.remove(item): Removes the first instance of the item.
- list.clear(): Removes all the items in the list
- list.reverse(): Reverses the elements in the list
- item in list: Checks if an item is present in the list (returns True or False).
Example 5: Iterating through a List
animals = ["dog", "cat", "bird"]
# Using a for loop with index
for i in range(len(animals)):
print(f"Index {i}: {animals[i]}")
print("\n") # New line
# Using a for loop more simply
for animal in animals:
print(f"Animal: {animal}")
Visualizing the For Loops
When using a for loop, it iterates over every element in the list:
for i in range(len(animals)):
- The loop goes through indexes: 0, 1, and 2
- animals[0] -> "dog"
- animals[1] -> "cat"
- animals[2] -> "bird"
- for animal in animals:
- The loop goes through elements directly
- animal -> "dog"
- animal -> "cat"
- animal -> "bird"
Let's Recap
- Arrays (represented by lists in Python) store collections of items.
- Each item has an index starting from 0.
- Lists in Python are flexible; you can easily modify them.
- You can access elements using their index (e.g., my_list[2]).
- Use methods like append(), insert(), pop(), and remove() to modify list content.
- Use len() to get the number of elements.
What's Your Reaction?
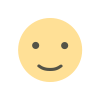
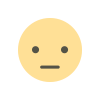
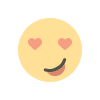
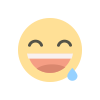
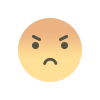
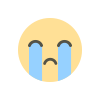
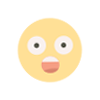