A Comprehensive Guide to useRouter() in Next.js 15
The useRouter hook is a powerful tool in Next.js, providing programmatic navigation and access to routing methods. With the release of Next.js 15, developers are encouraged to use useRouter from next/navigation, replacing the legacy next/router approach. This guide covers everything you need to know about useRouter in Next.js 15, including its features, usage, and best practices. Key Features of useRouter useRouter enables you to interact with the router object, providing functionality such as: Navigating to routes programmatically. Replacing or pushing entries in the history stack. Moving back or forward in the browser history. Prefetching routes to optimize performance. Refreshing the current page. Setting Up useRouter First, ensure you import useRouter from the correct package: import { useRouter } from 'next/navigation'; Note: The next/router package is deprecated in Next.js 15. Always use next/navigation for the latest features and best practices. Basic Navigation with useRouter Here’s an example of navigating programmatically to a different route: "use client"; import { useRouter } from 'next/navigation'; export default function HomePage() { const router = useRouter(); const goToAboutPage = () => { router.push('/about'); }; return ( Welcome to the Home Page Go to About Page ); } In this example: The router.push method navigates to the /about route without reloading the page. Advanced Routing Methods 1. push push adds a new entry to the browser history stack. This is useful for standard navigation. router.push('/profile'); When to Use: Navigating to a new page while keeping the ability to go back to the previous page. 2. replace replace updates the current history entry, effectively removing the previous entry. router.replace('/login'); When to Use: Redirecting users after a form submission or login, where going back to the previous page doesn’t make sense. 3. back back navigates to the previous page in the browser history. router.back(); When to Use: Providing a back button in your application to mimic browser behavior. 4. forward forward moves to the next page in the browser history stack (if available). router.forward(); When to Use: Useful in custom navigation where you want to replicate browser forward behavior. 5. refresh refresh reloads the current page without affecting the browser history. router.refresh(); When to Use: Re-fetching data or reloading the page to reflect the latest state. Prefetching Routes Next.js automatically prefetches routes for components, but you can also manually prefetch routes using router.prefetch: await router.prefetch('/dashboard'); When to Use: Preloading critical routes based on user behavior or patterns to improve perceived performance. Complete Example Here’s an example showcasing various useRouter methods: "use client"; import { useRouter } from 'next/navigation'; export default function NavigationExample() { const router = useRouter(); return ( router.push('/about')}>Go to About router.replace('/login')}>Redirect to Login router.back()}>Go Back router.forward()}>Go Forward router.refresh()}>Refresh Page ); } Best Practices 1. Use "use client" Appropriately Since useRouter is a client-side hook, add "use client" to the top of your file. Place it in small components like buttons or navigational elements to minimize unnecessary client-side rendering. 2. Optimize Navigation Logic Use replace for actions where going back is irrelevant, such as redirects. Use push for user-driven navigation, preserving the history stack. 3. Avoid Overusing refresh Rely on refresh only when necessary, as it reloads the entire page and can impact performance. 4. Combine with Dynamic Routes When working with dynamic routes, pass parameters programmatically: router.push(`/product/${productId}`); Deprecated vs. New Approach In older versions of Next.js, useRouter was imported from next/router. However, this is now deprecated in favor of next/navigation, which aligns with the latest App Router architecture. Conclusion The useRouter hook in Next.js 15 is a versatile and essential tool for building modern, client-side navigation. By leveraging its methods effectively, you can create intuitive, performant, and user-friendly applications. Whether you're navigating between pages, managing history, or prefetching routes, useRouter provides the flexibility to meet all your routing needs.

The useRouter
hook is a powerful tool in Next.js, providing programmatic navigation and access to routing methods. With the release of Next.js 15, developers are encouraged to use useRouter
from next/navigation
, replacing the legacy next/router
approach. This guide covers everything you need to know about useRouter
in Next.js 15, including its features, usage, and best practices.
Key Features of useRouter
useRouter
enables you to interact with the router object, providing functionality such as:
- Navigating to routes programmatically.
- Replacing or pushing entries in the history stack.
- Moving back or forward in the browser history.
- Prefetching routes to optimize performance.
- Refreshing the current page.
Setting Up useRouter
First, ensure you import useRouter
from the correct package:
import { useRouter } from 'next/navigation';
Note: The
next/router
package is deprecated in Next.js 15. Always usenext/navigation
for the latest features and best practices.
Basic Navigation with useRouter
Here’s an example of navigating programmatically to a different route:
"use client";
import { useRouter } from 'next/navigation';
export default function HomePage() {
const router = useRouter();
const goToAboutPage = () => {
router.push('/about');
};
return (
<div>
<h1>Welcome to the Home Pageh1>
<button onClick={goToAboutPage}>Go to About Pagebutton>
div>
);
}
In this example:
- The
router.push
method navigates to the/about
route without reloading the page.
Advanced Routing Methods
1. push
push
adds a new entry to the browser history stack. This is useful for standard navigation.
router.push('/profile');
- When to Use: Navigating to a new page while keeping the ability to go back to the previous page.
2. replace
replace
updates the current history entry, effectively removing the previous entry.
router.replace('/login');
- When to Use: Redirecting users after a form submission or login, where going back to the previous page doesn’t make sense.
3. back
back
navigates to the previous page in the browser history.
router.back();
- When to Use: Providing a back button in your application to mimic browser behavior.
4. forward
forward
moves to the next page in the browser history stack (if available).
router.forward();
- When to Use: Useful in custom navigation where you want to replicate browser forward behavior.
5. refresh
refresh
reloads the current page without affecting the browser history.
router.refresh();
- When to Use: Re-fetching data or reloading the page to reflect the latest state.
Prefetching Routes
Next.js automatically prefetches routes for components, but you can also manually prefetch routes using
router.prefetch
:
await router.prefetch('/dashboard');
- When to Use: Preloading critical routes based on user behavior or patterns to improve perceived performance.
Complete Example
Here’s an example showcasing various useRouter
methods:
"use client";
import { useRouter } from 'next/navigation';
export default function NavigationExample() {
const router = useRouter();
return (
<div>
<button onClick={() => router.push('/about')}>Go to Aboutbutton>
<button onClick={() => router.replace('/login')}>Redirect to Loginbutton>
<button onClick={() => router.back()}>Go Backbutton>
<button onClick={() => router.forward()}>Go Forwardbutton>
<button onClick={() => router.refresh()}>Refresh Pagebutton>
div>
);
}
Best Practices
1. Use "use client"
Appropriately
Since useRouter
is a client-side hook, add "use client"
to the top of your file. Place it in small components like buttons or navigational elements to minimize unnecessary client-side rendering.
2. Optimize Navigation Logic
- Use
replace
for actions where going back is irrelevant, such as redirects. - Use
push
for user-driven navigation, preserving the history stack.
3. Avoid Overusing refresh
Rely on refresh
only when necessary, as it reloads the entire page and can impact performance.
4. Combine with Dynamic Routes
When working with dynamic routes, pass parameters programmatically:
router.push(`/product/${productId}`);
Deprecated vs. New Approach
In older versions of Next.js, useRouter
was imported from next/router
. However, this is now deprecated in favor of next/navigation
, which aligns with the latest App Router architecture.
Conclusion
The useRouter
hook in Next.js 15 is a versatile and essential tool for building modern, client-side navigation. By leveraging its methods effectively, you can create intuitive, performant, and user-friendly applications. Whether you're navigating between pages, managing history, or prefetching routes, useRouter
provides the flexibility to meet all your routing needs.
What's Your Reaction?
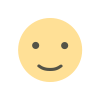
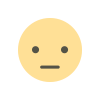
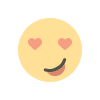
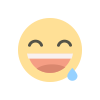
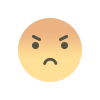
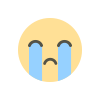
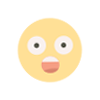