Why is the Python crawler running so slowly? How to optimize it?
In the development process of Python crawler, low operating efficiency is a common and troublesome problem. This article will explore the reasons why Python crawler runs slowly and provide a series of practical optimization strategies to help developers significantly improve the running speed of crawlers. At the same time, we will also mention 98IP proxy as one of the optimization methods to further improve crawler performance. I. Analysis of the reasons why Python crawler runs slowly 1.1 Inefficient network request Network request is a key link in the operation of crawler, but it is also the most likely place for bottlenecks. The reasons may include: Frequent HTTP requests: If the crawler frequently sends HTTP requests without proper merging or scheduling, it will cause frequent network IO operations, thereby slowing down the overall speed. Improper request interval: Too short request interval may trigger the anti-crawler mechanism of the target website, resulting in request blocking or IP being banned, thereby increasing the number of retries and reducing efficiency. 1.2 Data processing bottleneck Data processing is another major overhead of crawlers, especially when processing large amounts of data. The reasons may include: Complex data parsing: Using inefficient data parsing methods, such as regular expressions (regex) to process complex HTML structures, will significantly affect the processing speed. Improper memory management: Loading a large amount of data into memory at one time not only takes up a lot of resources, but may also cause memory leaks and affect system performance. 1.3 Unreasonable concurrency control Concurrency control is an important means to improve crawler efficiency, but if it is unreasonable, it may reduce efficiency. The reasons may include: Improper thread/process management: Failure to fully utilize multi-core CPU resources, or excessive communication overhead between threads/processes, resulting in the inability to play the concurrency advantage. Improper asynchronous programming: When using asynchronous programming, if the event loop design is unreasonable or the task scheduling is improper, it will lead to performance bottlenecks. II. Python crawler optimization strategy 2.1 Optimize network requests Use efficient HTTP libraries: such as requests libraries, which are more efficient than urllib and support connection pools, which can reduce the overhead of TCP connections. Merge requests: For requests that can be merged, try to merge them to reduce the number of network IOs. Set a reasonable request interval: avoid too short request intervals to prevent triggering anti-crawler mechanisms. You can use the time.sleep() function to set the request interval. 2.2 Optimize data processing Use efficient parsing methods: such as using BeautifulSoup or lxml libraries to parse HTML, which are more efficient than regular expressions. Process data in batches: do not load all data into memory at once, but process in batches to reduce memory usage. Use generators: generators can generate data on demand, avoid loading all data into memory at once, and improve memory utilization. 2.3 Optimize concurrency control Use multi-threading/multi-processes: reasonably allocate the number of threads/processes according to the number of CPU cores, and make full use of multi-core CPU resources. Use asynchronous programming: such as the asyncio library, which allows concurrent tasks to be executed in a single thread, reducing the communication overhead between threads/processes. Use task queues: such as concurrent.futures.ThreadPoolExecutor or ProcessPoolExecutor, which can manage task queues and automatically schedule tasks. 2.4 Use proxy IP (take 98IP proxy as an example) Avoid IP blocking: Using proxy IP can hide the real IP address and prevent the crawler from being blocked by the target website. Especially when frequently visiting the same website, using proxy IP can significantly reduce the risk of being blocked. Increase request success rate: By changing the proxy IP, you can bypass the geographical restrictions or access restrictions of certain websites and increase the request success rate. This is especially useful for visiting foreign websites or websites that require IP access from a specific region. 98IP proxy service: 98IP proxy provides high-quality proxy IP resources and supports multiple protocols and regional options. Using 98IP proxy can significantly improve crawler performance while reducing the risk of being blocked. When using it, just configure the proxy IP to the proxy settings of the HTTP request. III. Sample code The following is a sample code that uses the requests library and BeautifulSoup library to crawl web pages, uses concurrent.futures.ThreadPoolExecutor for concurrency control, and configures 98IP proxy: import requ

In the development process of Python crawler, low operating efficiency is a common and troublesome problem. This article will explore the reasons why Python crawler runs slowly and provide a series of practical optimization strategies to help developers significantly improve the running speed of crawlers. At the same time, we will also mention 98IP proxy as one of the optimization methods to further improve crawler performance.
I. Analysis of the reasons why Python crawler runs slowly
1.1 Inefficient network request
Network request is a key link in the operation of crawler, but it is also the most likely place for bottlenecks. The reasons may include:
- Frequent HTTP requests: If the crawler frequently sends HTTP requests without proper merging or scheduling, it will cause frequent network IO operations, thereby slowing down the overall speed.
- Improper request interval: Too short request interval may trigger the anti-crawler mechanism of the target website, resulting in request blocking or IP being banned, thereby increasing the number of retries and reducing efficiency.
1.2 Data processing bottleneck
Data processing is another major overhead of crawlers, especially when processing large amounts of data. The reasons may include:
- Complex data parsing: Using inefficient data parsing methods, such as regular expressions (regex) to process complex HTML structures, will significantly affect the processing speed.
- Improper memory management: Loading a large amount of data into memory at one time not only takes up a lot of resources, but may also cause memory leaks and affect system performance.
1.3 Unreasonable concurrency control
Concurrency control is an important means to improve crawler efficiency, but if it is unreasonable, it may reduce efficiency. The reasons may include:
- Improper thread/process management: Failure to fully utilize multi-core CPU resources, or excessive communication overhead between threads/processes, resulting in the inability to play the concurrency advantage.
- Improper asynchronous programming: When using asynchronous programming, if the event loop design is unreasonable or the task scheduling is improper, it will lead to performance bottlenecks.
II. Python crawler optimization strategy
2.1 Optimize network requests
-
Use efficient HTTP libraries: such as
requests
libraries, which are more efficient thanurllib
and support connection pools, which can reduce the overhead of TCP connections. - Merge requests: For requests that can be merged, try to merge them to reduce the number of network IOs.
-
Set a reasonable request interval: avoid too short request intervals to prevent triggering anti-crawler mechanisms. You can use the
time.sleep()
function to set the request interval.
2.2 Optimize data processing
-
Use efficient parsing methods: such as using
BeautifulSoup
orlxml
libraries to parse HTML, which are more efficient than regular expressions. - Process data in batches: do not load all data into memory at once, but process in batches to reduce memory usage.
- Use generators: generators can generate data on demand, avoid loading all data into memory at once, and improve memory utilization.
2.3 Optimize concurrency control
- Use multi-threading/multi-processes: reasonably allocate the number of threads/processes according to the number of CPU cores, and make full use of multi-core CPU resources.
-
Use asynchronous programming: such as the
asyncio
library, which allows concurrent tasks to be executed in a single thread, reducing the communication overhead between threads/processes. -
Use task queues: such as
concurrent.futures.ThreadPoolExecutor
orProcessPoolExecutor
, which can manage task queues and automatically schedule tasks.
2.4 Use proxy IP (take 98IP proxy as an example)
- Avoid IP blocking: Using proxy IP can hide the real IP address and prevent the crawler from being blocked by the target website. Especially when frequently visiting the same website, using proxy IP can significantly reduce the risk of being blocked.
- Increase request success rate: By changing the proxy IP, you can bypass the geographical restrictions or access restrictions of certain websites and increase the request success rate. This is especially useful for visiting foreign websites or websites that require IP access from a specific region.
- 98IP proxy service: 98IP proxy provides high-quality proxy IP resources and supports multiple protocols and regional options. Using 98IP proxy can significantly improve crawler performance while reducing the risk of being blocked. When using it, just configure the proxy IP to the proxy settings of the HTTP request.
III. Sample code
The following is a sample code that uses the requests
library and BeautifulSoup
library to crawl web pages, uses concurrent.futures.ThreadPoolExecutor
for concurrency control, and configures 98IP proxy:
import requests
from bs4 import BeautifulSoup
from concurrent.futures import ThreadPoolExecutor
# Target URL List
urls = [
'http://example.com/page1',
'http://example.com/page2',
# .... More URLs
]
# 98IP Proxy Configuration (example, need to replace with a valid 98IP proxy for actual use)
proxy = 'http://your_98ip_proxy:port' # Replace it with your 98 IP proxy address and port
# Crawl Functions
def fetch_page(url):
try:
headers = {'User-Agent': 'Mozilla/5.0'}
proxies = {'http': proxy, 'https': proxy}
response = requests.get(url, headers=headers, proxies=proxies)
response.raise_for_status() # Check if the request was successful
soup = BeautifulSoup(response.text, 'html.parser')
# The parsed data is processed here
print(soup.title.string) # Print the page title as an example
except Exception as e:
print(f"Error fetching {url}: {e}")
# Concurrency Control with ThreadPoolExecutor
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(fetch_page, urls)
In the above code, we use ThreadPoolExecutor
to manage the thread pool and set a maximum of 5 working threads. Each thread calls the fetch_page
function to crawl the specified URL. In the fetch_page
function, we use the requests
library to send HTTP requests and configure the 98IP proxy to hide the real IP address. At the same time, we also use the BeautifulSoup
library to parse the HTML content and print the page title as an example.
IV. Summary
The reasons for the slow operation of Python crawlers may involve network requests, data processing, and concurrency control. By optimizing these aspects, we can significantly improve the running speed of the crawler. In addition, using proxy IP is also one of the important means to improve the performance of the crawler. As a high-quality proxy IP service provider, 98IP proxy can significantly improve the performance of the crawler and reduce the risk of being banned. I hope that the content of this article can help developers better understand and optimize the performance of Python crawlers.
What's Your Reaction?
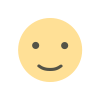
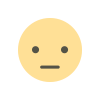
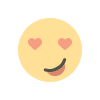
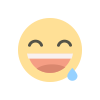
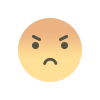
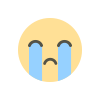
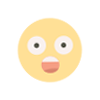