Why I Love: git reset --hard
Managing version control is both an art and a science, and for many developers, Git is the tool of choice. While Git offers a plethora of commands to handle almost any situation, there is one command I hold dear to my heart: git reset --hard. It’s my go-to solution when things go haywire, and it’s the unsung hero that has saved me countless times. In this article, I’ll explain why I love it, how it makes my life easier, and how you can use it effectively. What Does git reset --hard Do? At its core, git reset --hard is about resetting your Git repository to a clean state. When you run this command, three things happen: HEAD Moves: The HEAD pointer is moved to a specific commit. Staging Area Reset: The staging area is updated to match the target commit. Working Directory Reset: All uncommitted changes in your working directory are discarded to match the target commit. In simpler terms, it’s like hitting a "factory reset" button for your Git repository. Here’s the syntax: git reset --hard If you omit the commit hash, it defaults to the latest commit. Why git reset --hard Is a Game-Changer 1. Undoing Mistakes Instantly There have been countless times when I made experimental changes that didn’t pan out. Instead of manually reverting each file or trying to stash changes, I simply run: git reset --hard Within seconds, my repository is back to its last stable state. It’s quick, efficient, and hassle-free. 2. Getting a Clean Slate When working on large projects, the working directory can get cluttered with temporary changes. Sometimes, you just want to start fresh. Example scenario: You’re debugging an issue and have tried multiple approaches. None of them worked, and your directory is now a mess of temporary files and edits. Instead of sifting through the debris, git reset --hard wipes the slate clean, letting you start anew. 3. Recovering from Merge Chaos Merges don’t always go as planned, and conflicts can leave your repository in an inconsistent state. When I realize a merge has gone sideways, I use: git reset --hard This resets my repository to a pre-merge state, allowing me to approach the merge more carefully. 4. Experimentation Without Fear One of the joys of being a developer is experimenting with new ideas. But experiments often lead to dead ends. Knowing I can always reset my repository gives me the freedom to try new things without worrying about breaking anything permanently. Use It, But Use It Wisely As powerful as git reset --hard is, it comes with a caveat: it’s irreversible. Once you run this command, any uncommitted changes are gone for good. To avoid losing important work, here are some tips: Check the Commit History: Use git log to ensure you’re resetting to the right commit. git log --oneline Use git reflog for Recovery: If you accidentally reset to the wrong commit, git reflog can help you find your previous HEAD position. git reflog git reset --hard Commit or Stash First: Before running git reset --hard, always commit or stash any changes you might need later. git stash save "WIP changes" Caution: The Double-Edged Sword git reset --hard is incredibly powerful, but with great power comes great responsibility. It permanently discards uncommitted changes, which means there’s no way to recover them unless you’ve stashed or committed first. Always double-check your changes and use commands like git log or git reflog to ensure you’re targeting the correct commit. This cautionary step can save you from accidental data loss. Real-World Examples 1. Fixing a Botched Feature Imagine you’re halfway through implementing a new feature, and it’s not working as expected. You realize it’d be faster to start over. Here’s what you do: git reset --hard In seconds, you’re back to where you started, ready to tackle the feature with a fresh perspective. 2. Cleaning Up After a Failed Rebase Rebasing can sometimes lead to unexpected conflicts or an incomplete history. If a rebase goes wrong, you can use: git reset --hard ORIG_HEAD This resets your branch to its original state before the rebase. Why I Love It git reset --hard is more than just a command; it’s a safety net. It gives me the confidence to experiment, the ability to recover quickly, and the freedom to focus on solving problems without worrying about making mistakes. It’s a tool that’s simple yet incredibly powerful. If you haven’t already embraced git reset --hard, I encourage you to give it a try. Just remember to use it wisely, and it’ll become one of your favorite Git commands too. Final Thoughts Version control is an essential skill for developers, and mastering commands like git reset --hard can make your life significantly easier. While it may seem intimidating at first,

Managing version control is both an art and a science, and for many developers, Git is the tool of choice. While Git offers a plethora of commands to handle almost any situation, there is one command I hold dear to my heart: git reset --hard
. It’s my go-to solution when things go haywire, and it’s the unsung hero that has saved me countless times. In this article, I’ll explain why I love it, how it makes my life easier, and how you can use it effectively.
What Does git reset --hard
Do?
At its core, git reset --hard
is about resetting your Git repository to a clean state. When you run this command, three things happen:
- HEAD Moves: The HEAD pointer is moved to a specific commit.
- Staging Area Reset: The staging area is updated to match the target commit.
- Working Directory Reset: All uncommitted changes in your working directory are discarded to match the target commit.
In simpler terms, it’s like hitting a "factory reset" button for your Git repository. Here’s the syntax:
git reset --hard
If you omit the commit hash, it defaults to the latest commit.
Why git reset --hard
Is a Game-Changer
1. Undoing Mistakes Instantly
There have been countless times when I made experimental changes that didn’t pan out. Instead of manually reverting each file or trying to stash changes, I simply run:
git reset --hard
Within seconds, my repository is back to its last stable state. It’s quick, efficient, and hassle-free.
2. Getting a Clean Slate
When working on large projects, the working directory can get cluttered with temporary changes. Sometimes, you just want to start fresh.
Example scenario:
- You’re debugging an issue and have tried multiple approaches.
- None of them worked, and your directory is now a mess of temporary files and edits.
Instead of sifting through the debris, git reset --hard
wipes the slate clean, letting you start anew.
3. Recovering from Merge Chaos
Merges don’t always go as planned, and conflicts can leave your repository in an inconsistent state. When I realize a merge has gone sideways, I use:
git reset --hard
This resets my repository to a pre-merge state, allowing me to approach the merge more carefully.
4. Experimentation Without Fear
One of the joys of being a developer is experimenting with new ideas. But experiments often lead to dead ends. Knowing I can always reset my repository gives me the freedom to try new things without worrying about breaking anything permanently.
Use It, But Use It Wisely
As powerful as git reset --hard
is, it comes with a caveat: it’s irreversible. Once you run this command, any uncommitted changes are gone for good. To avoid losing important work, here are some tips:
-
Check the Commit History:
Use
git log
to ensure you’re resetting to the right commit.
git log --oneline
-
Use
git reflog
for Recovery: If you accidentally reset to the wrong commit,git reflog
can help you find your previous HEAD position.
git reflog
git reset --hard
-
Commit or Stash First:
Before running
git reset --hard
, always commit or stash any changes you might need later.
git stash save "WIP changes"
Caution: The Double-Edged Sword
git reset --hard
is incredibly powerful, but with great power comes great responsibility. It permanently discards uncommitted changes, which means there’s no way to recover them unless you’ve stashed or committed first. Always double-check your changes and use commands like git log
or git reflog
to ensure you’re targeting the correct commit. This cautionary step can save you from accidental data loss.
Real-World Examples
1. Fixing a Botched Feature
Imagine you’re halfway through implementing a new feature, and it’s not working as expected. You realize it’d be faster to start over. Here’s what you do:
git reset --hard
In seconds, you’re back to where you started, ready to tackle the feature with a fresh perspective.
2. Cleaning Up After a Failed Rebase
Rebasing can sometimes lead to unexpected conflicts or an incomplete history. If a rebase goes wrong, you can use:
git reset --hard ORIG_HEAD
This resets your branch to its original state before the rebase.
Why I Love It
git reset --hard
is more than just a command; it’s a safety net. It gives me the confidence to experiment, the ability to recover quickly, and the freedom to focus on solving problems without worrying about making mistakes. It’s a tool that’s simple yet incredibly powerful.
If you haven’t already embraced git reset --hard
, I encourage you to give it a try. Just remember to use it wisely, and it’ll become one of your favorite Git commands too.
Final Thoughts
Version control is an essential skill for developers, and mastering commands like git reset --hard
can make your life significantly easier. While it may seem intimidating at first, understanding its nuances will empower you to handle even the messiest of repositories with confidence. For me, it’s an indispensable part of my workflow, and I hope it can be for you too.
What's Your Reaction?
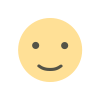
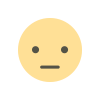
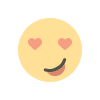
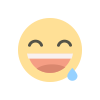
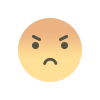
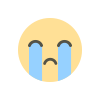
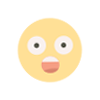