TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type
TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type TypeScript is a powerful programming language that builds on JavaScript by adding static type definitions. This means you can define types for variables, function parameters, and return values, enhancing the reliability of your code. Types in TypeScript help catch errors during development, making your code easier to understand and maintain. If you're eager to dive deeper into TypeScript or learn how to utilize AI tools like gpteach to code, I highly recommend subscribing to my blog! You can also join gpteach at gpteach.us for more coding insights. Understanding Types in TypeScript In TypeScript, types are a way to define the nature of data that can be stored and manipulated in your program. They can be simple types like string, number, or boolean, or more complex constructs like interfaces, enums, and unions. Here's a quick example of defining a type: let userName: string = "Alice"; let userAge: number = 30; let isActive: boolean = true; With these definitions, TypeScript knows the kind of data each variable can hold, which helps catch errors before runtime. What is a Superset Language? A superset language is a programming language that extends the functionalities of another language. TypeScript is a superset of JavaScript, which means that all valid JavaScript code is also valid TypeScript code. This allows developers to gradually adopt TypeScript in their JavaScript projects without needing to rewrite everything from scratch. TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type Now, let’s delve into one specific TypeScript error: TS1166. This error occurs when you use computed property names in class property declarations, but the computed property does not resolve to a simple literal type or a unique symbol type. In TypeScript, computed property names are used to define object properties dynamically. However, when you declare a class property using a computed property name, the result must be either a simple literal (like a string or number) or a unique symbol. Example of the Error Here is an example that triggers TS1166: class MyClass { [key: string]: any; // This will cause TS1166 } In this case, using key: string does not satisfy the requirements of TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type. How to Fix the Error To resolve this error, you can use a simple string literal or a unique symbol type. Here’s how you can modify the code: const myKey = "propName"; class MyClass { [myKey]: number; // Fixed: Using a simple literal type } In this updated example, myKey is a string literal, thus satisfying the requirements outlined by TS1166. Important to Know! Simple Literal Types: These include primitive data types like string, number, and boolean. Always ensure computed property names resolve to these types to avoid TS1166. Unique Symbols: You can create unique symbols in TypeScript using the Symbol() function, allowing for unique property keys that can also be used as computed property names. Here’s an example using a unique symbol: const mySymbolKey = Symbol("uniqueKey"); class MyClass { [mySymbolKey]: boolean; // This is valid as it uses a unique symbol type } FAQ Section Q: Why does TypeScript distinguish between simple literal types and other types? A: This distinction helps maintain consistency and predictability when accessing property values. It prevents potential errors that could occur from using more complex or dynamic types. Q: Can I use other data types for computed property names? A: No, according to TS1166, you must use simple literal types or unique symbols for computed property names in class declarations. Q: How can I check for these types in my code? A: You can use TypeScript's type-checking features which provide immediate feedback during development, enhancing code quality. Summary In summary, understanding the error message TS1166 is crucial for TypeScript developers, especially when utilizing computed property names in class property declarations. Make sure to use simple literal types or unique symbols to avoid this error, and you'll keep your TypeScript code tidy and functional. If you're looking to further your understanding of TypeScript and catch common errors like TS1166 as you write code, consider checking out gpteach.us for insightful resources and coding education. Happy coding!

TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type
TypeScript is a powerful programming language that builds on JavaScript by adding static type definitions. This means you can define types for variables, function parameters, and return values, enhancing the reliability of your code. Types in TypeScript help catch errors during development, making your code easier to understand and maintain.
If you're eager to dive deeper into TypeScript or learn how to utilize AI tools like gpteach to code, I highly recommend subscribing to my blog! You can also join gpteach at gpteach.us for more coding insights.
Understanding Types in TypeScript
In TypeScript, types are a way to define the nature of data that can be stored and manipulated in your program. They can be simple types like string
, number
, or boolean
, or more complex constructs like interfaces, enums, and unions. Here's a quick example of defining a type:
let userName: string = "Alice";
let userAge: number = 30;
let isActive: boolean = true;
With these definitions, TypeScript knows the kind of data each variable can hold, which helps catch errors before runtime.
What is a Superset Language?
A superset language is a programming language that extends the functionalities of another language. TypeScript is a superset of JavaScript, which means that all valid JavaScript code is also valid TypeScript code. This allows developers to gradually adopt TypeScript in their JavaScript projects without needing to rewrite everything from scratch.
TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type
Now, let’s delve into one specific TypeScript error: TS1166. This error occurs when you use computed property names in class property declarations, but the computed property does not resolve to a simple literal type or a unique symbol type.
In TypeScript, computed property names are used to define object properties dynamically. However, when you declare a class property using a computed property name, the result must be either a simple literal (like a string or number) or a unique symbol.
Example of the Error
Here is an example that triggers TS1166:
class MyClass {
[key: string]: any; // This will cause TS1166
}
In this case, using key: string
does not satisfy the requirements of TS1166: A computed property name in a class property declaration must have a simple literal type or a 'unique symbol' type.
How to Fix the Error
To resolve this error, you can use a simple string literal or a unique symbol type. Here’s how you can modify the code:
const myKey = "propName";
class MyClass {
[myKey]: number; // Fixed: Using a simple literal type
}
In this updated example, myKey
is a string literal, thus satisfying the requirements outlined by TS1166.
Important to Know!
Simple Literal Types: These include primitive data types like
string
,number
, andboolean
. Always ensure computed property names resolve to these types to avoid TS1166.Unique Symbols: You can create unique symbols in TypeScript using the
Symbol()
function, allowing for unique property keys that can also be used as computed property names.
Here’s an example using a unique symbol:
const mySymbolKey = Symbol("uniqueKey");
class MyClass {
[mySymbolKey]: boolean; // This is valid as it uses a unique symbol type
}
FAQ Section
Q: Why does TypeScript distinguish between simple literal types and other types?
A: This distinction helps maintain consistency and predictability when accessing property values. It prevents potential errors that could occur from using more complex or dynamic types.
Q: Can I use other data types for computed property names?
A: No, according to TS1166, you must use simple literal types or unique symbols for computed property names in class declarations.
Q: How can I check for these types in my code?
A: You can use TypeScript's type-checking features which provide immediate feedback during development, enhancing code quality.
Summary
In summary, understanding the error message TS1166 is crucial for TypeScript developers, especially when utilizing computed property names in class property declarations. Make sure to use simple literal types or unique symbols to avoid this error, and you'll keep your TypeScript code tidy and functional.
If you're looking to further your understanding of TypeScript and catch common errors like TS1166 as you write code, consider checking out gpteach.us for insightful resources and coding education. Happy coding!
What's Your Reaction?
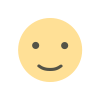
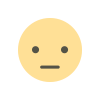
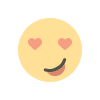
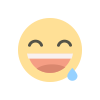
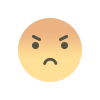
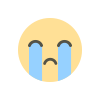
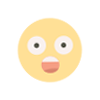