Overview of RESTful API Development: A Task-Based Journey
Overview of RESTful API Development: A Task-Based Journey Introduction Recently, I embarked on a task to build a RESTful API as part of my #100DaysOfCode challenge. This journey not only strengthened my understanding of API development but also highlighted some common pitfalls, particularly around database connection strings. Here’s a detailed overview of my experience. Getting Started with RESTful APIs To kick off the project, I aimed to create a simple RESTful API using Express.js and MongoDB. The goal was to handle basic CRUD operations effectively. Setting Up the Environment Project Initialization: I installed Node.js and initialized a new project using npm init. Essential packages like Express and Mongoose were added: npm install express mongoose dotenv Creating the Server: A basic Express server was set up in server.js, with middleware configured to parse JSON request bodies: app.use(express.json()); The Connection String Conundrum One of the key challenges I faced was with the MongoDB connection string. Initially, I used placeholders like username and password, which is a common mistake. Here’s a snippet of what that looked like: mongodb+srv://username:password@cluster.mongodb.net/myDatabase?retryWrites=true&w=majority It’s crucial to replace these placeholders with actual credentials, ensuring the connection string is properly formatted. I encountered authentication errors during testing, which led me to realize that I had omitted the necessary details in the string. This oversight prevented my application from connecting to the database, causing unnecessary delays in my development process. Connecting to MongoDB After properly updating the connection string in my .env file, I successfully connected to my MongoDB Atlas database using Mongoose. This step was vital for ensuring that my application could store and retrieve data effectively. Defining Routes With the server up and running, I defined routes to handle CRUD operations: GET /api/items: Fetches all items from the database. POST /api/items: Adds a new item to the database. PUT /api/items/:id: Updates an existing item by ID. DELETE /api/items/:id: Deletes an item by ID. Implementing Error Handling I implemented error handling to manage various scenarios, such as invalid requests or missing parameters. This was essential for providing clear feedback to users and ensuring a smoother experience. Data Validation To enhance data integrity, I used validation libraries to ensure that incoming data met specific criteria. This step was crucial in preventing bad data from being processed by the API. Testing the API After completing the API, I conducted thorough testing using Postman. This tool allowed me to simulate various requests to the API endpoints and verify their functionality. I pushed my code to GitHub, making it easier to track changes and collaborate if needed. Deployment to Render Once I was satisfied with the API's performance in testing, I deployed it to Render. This platform simplifies the deployment process for web applications and automatically handles scaling. To access the API, I set the root route to respond with a message indicating that the server was running. This is a common practice to confirm that the API is live and functioning correctly. Here's an example of how I implemented this: app.get('/', (req, res) => { res.send('welcome to restful Api!'); }); Conclusion Building this RESTful API has been a rewarding task that reinforced my understanding of web development. The process highlighted the importance of careful configuration, especially when dealing with connection strings. Key Takeaways Always ensure that your MongoDB connection string is correctly formatted, replacing placeholders like username and password with actual credentials. Understanding CRUD operations is essential for effective API design. Robust error handling and data validation are critical for building reliable applications. Using tools like Postman for testing and platforms like Render for deployment can streamline your development workflow. This experience has not only improved my coding skills but also prepared me for future projects in the ever-evolving world of web development. I look forward to sharing more insights and continuing my journey!
Overview of RESTful API Development: A Task-Based Journey
Introduction
Recently, I embarked on a task to build a RESTful API as part of my #100DaysOfCode challenge. This journey not only strengthened my understanding of API development but also highlighted some common pitfalls, particularly around database connection strings. Here’s a detailed overview of my experience.
Getting Started with RESTful APIs
To kick off the project, I aimed to create a simple RESTful API using Express.js and MongoDB. The goal was to handle basic CRUD operations effectively.
Setting Up the Environment
-
Project Initialization:
- I installed Node.js and initialized a new project using
npm init
. - Essential packages like Express and Mongoose were added:
npm install express mongoose dotenv
- I installed Node.js and initialized a new project using
-
Creating the Server:
- A basic Express server was set up in
server.js
, with middleware configured to parse JSON request bodies:
app.use(express.json());
- A basic Express server was set up in
The Connection String Conundrum
One of the key challenges I faced was with the MongoDB connection string. Initially, I used placeholders like username
and password
, which is a common mistake. Here’s a snippet of what that looked like:
mongodb+srv://username:password@cluster.mongodb.net/myDatabase?retryWrites=true&w=majority
It’s crucial to replace these placeholders with actual credentials, ensuring the connection string is properly formatted. I encountered authentication errors during testing, which led me to realize that I had omitted the necessary details in the string. This oversight prevented my application from connecting to the database, causing unnecessary delays in my development process.
Connecting to MongoDB
After properly updating the connection string in my .env
file, I successfully connected to my MongoDB Atlas database using Mongoose. This step was vital for ensuring that my application could store and retrieve data effectively.
Defining Routes
With the server up and running, I defined routes to handle CRUD operations:
- GET /api/items: Fetches all items from the database.
- POST /api/items: Adds a new item to the database.
- PUT /api/items/:id: Updates an existing item by ID.
- DELETE /api/items/:id: Deletes an item by ID.
Implementing Error Handling
I implemented error handling to manage various scenarios, such as invalid requests or missing parameters. This was essential for providing clear feedback to users and ensuring a smoother experience.
Data Validation
To enhance data integrity, I used validation libraries to ensure that incoming data met specific criteria. This step was crucial in preventing bad data from being processed by the API.
Testing the API
After completing the API, I conducted thorough testing using Postman. This tool allowed me to simulate various requests to the API endpoints and verify their functionality. I pushed my code to GitHub, making it easier to track changes and collaborate if needed.
Deployment to Render
Once I was satisfied with the API's performance in testing, I deployed it to Render. This platform simplifies the deployment process for web applications and automatically handles scaling.
To access the API, I set the root route to respond with a message indicating that the server was running. This is a common practice to confirm that the API is live and functioning correctly. Here's an example of how I implemented this:
app.get('/', (req, res) => {
res.send('welcome to restful Api!');
});
Conclusion
Building this RESTful API has been a rewarding task that reinforced my understanding of web development. The process highlighted the importance of careful configuration, especially when dealing with connection strings.
Key Takeaways
- Always ensure that your MongoDB connection string is correctly formatted, replacing placeholders like
username
andpassword
with actual credentials. - Understanding CRUD operations is essential for effective API design.
- Robust error handling and data validation are critical for building reliable applications.
- Using tools like Postman for testing and platforms like Render for deployment can streamline your development workflow.
This experience has not only improved my coding skills but also prepared me for future projects in the ever-evolving world of web development. I look forward to sharing more insights and continuing my journey!
What's Your Reaction?
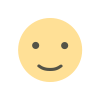
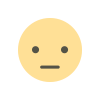
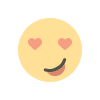
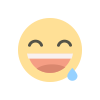
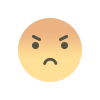
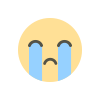
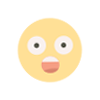