Nextjs Dockerfile
Nextjs Dockerfile is the key for Building and Running a Next.js Application with Docker. Docker simplifies the process of deploying and running Next.js applications by creating a consistent environment across development, staging, and production. Building and Running a Next.js Application with Docker Below, we explore a robust Dockerfile for building and deploying a Next.js application. This setup ensures optimal performance and flexibility. Dockerfile Explanation Here’s a detailed explanation of the provided Dockerfile by Vercel Github Page: # syntax=docker.io/docker/dockerfile:1 FROM node:18-alpine AS base # Install dependencies only when needed FROM base AS deps # Check https://github.com/nodejs/docker-node/tree/b4117f9333da4138b03a546ec926ef50a31506c3#nodealpine to understand why libc6-compat might be needed. RUN apk add --no-cache libc6-compat WORKDIR /app # Install dependencies based on the preferred package manager COPY package.json yarn.lock* package-lock.json* pnpm-lock.yaml* .npmrc* ./ RUN \ if [ -f yarn.lock ]; then yarn --frozen-lockfile; \ elif [ -f package-lock.json ]; then npm ci; \ elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm i --frozen-lockfile; \ else echo "Lockfile not found." && exit 1; \ fi # Rebuild the source code only when needed FROM base AS builder WORKDIR /app COPY --from=deps /app/node_modules ./node_modules COPY . . # Disable telemetry if desired # ENV NEXT_TELEMETRY_DISABLED=1 RUN \ if [ -f yarn.lock ]; then yarn run build; \ elif [ -f package-lock.json ]; then npm run build; \ elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm run build; \ else echo "Lockfile not found." && exit 1; \ fi # Production image, copy all the files and run Next.js FROM base AS runner WORKDIR /app ENV NODE_ENV=production # Disable telemetry during runtime if desired # ENV NEXT_TELEMETRY_DISABLED=1 RUN addgroup --system --gid 1001 nodejs RUN adduser --system --uid 1001 nextjs COPY --from=builder /app/public ./public # Leverage output traces to reduce image size COPY --from=builder --chown=nextjs:nodejs /app/.next/standalone ./ COPY --from=builder --chown=nextjs:nodejs /app/.next/static ./.next/static USER nextjs EXPOSE 3000 ENV PORT=3000 ENV HOSTNAME="0.0.0.0" # Start the Next.js server CMD ["node", "server.js"] Phases of the Docker Build Process 1. Base Image (base stage) The node:18-alpine image is used for a lightweight Node.js runtime. libc6-compat is installed to resolve compatibility issues with Alpine-based images. 2. Dependency Installation (deps stage) Dependency installation is based on the detected lockfile (yarn.lock, package-lock.json, or pnpm-lock.yaml). Ensures consistency by locking dependencies. 3. Application Build (builder stage) Copies project files and dependencies into the container. Builds the Next.js application using yarn, npm, or pnpm, depending on the lockfile. Outputs a production-ready standalone build. 4. Production Runner (runner stage) Configures a production-ready environment. Creates a non-root user (nextjs) to enhance security. Copies the necessary output (public, .next/standalone, and .next/static) from the build stage. Exposes port 3000 and starts the Next.js server. Key Features 1. Multi-Stage Builds Separates the build process into distinct stages to optimize image size and security. Ensures that the production image contains only the necessary runtime files. 2. Flexible Dependency Management Detects the appropriate package manager based on the lockfile. Ensures compatibility with different teams' preferences (Yarn, npm, pnpm). 3. Security Best Practices Runs the application as a non-root user (nextjs). Uses the alpine base image for a minimal attack surface. 4. Reduced Image Size Utilizes Next.js output file tracing to include only essential files in the production image. Leverages .next/standalone for optimized deployments. Running the Dockerized Next.js Application Build the Image Run the following command to build the Docker image: docker build -t nextjs-app . Run the Container Start the container using: docker run -p 3000:3000 nextjs-app Access the application at http://localhost:3000. Customizations Disable Telemetry: Uncomment NEXT_TELEMETRY_DISABLED=1 to disable anonymous telemetry data collection during build and runtime. Environment Variables: Add environment-specific configurations using ENV directives or .env files. Conclusion This Nextjs Dockerfile provides a comprehensive and efficient way to build and deploy Next.js applications. By leveraging multi-stage builds, output tracing, and best practices for security and performance, you can confidently deploy your Next.js projects in production environments. Adapt the Dockerfile to

Nextjs Dockerfile is the key for Building and Running a Next.js Application with Docker. Docker simplifies the process of deploying and running Next.js applications by creating a consistent environment across development, staging, and production.
Building and Running a Next.js Application with Docker
Below, we explore a robust Dockerfile
for building and deploying a Next.js application. This setup ensures optimal performance and flexibility.
Dockerfile Explanation
Here’s a detailed explanation of the provided Dockerfile
by Vercel Github Page:
# syntax=docker.io/docker/dockerfile:1
FROM node:18-alpine AS base
# Install dependencies only when needed
FROM base AS deps
# Check https://github.com/nodejs/docker-node/tree/b4117f9333da4138b03a546ec926ef50a31506c3#nodealpine to understand why libc6-compat might be needed.
RUN apk add --no-cache libc6-compat
WORKDIR /app
# Install dependencies based on the preferred package manager
COPY package.json yarn.lock* package-lock.json* pnpm-lock.yaml* .npmrc* ./
RUN \
if [ -f yarn.lock ]; then yarn --frozen-lockfile; \
elif [ -f package-lock.json ]; then npm ci; \
elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm i --frozen-lockfile; \
else echo "Lockfile not found." && exit 1; \
fi
# Rebuild the source code only when needed
FROM base AS builder
WORKDIR /app
COPY --from=deps /app/node_modules ./node_modules
COPY . .
# Disable telemetry if desired
# ENV NEXT_TELEMETRY_DISABLED=1
RUN \
if [ -f yarn.lock ]; then yarn run build; \
elif [ -f package-lock.json ]; then npm run build; \
elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm run build; \
else echo "Lockfile not found." && exit 1; \
fi
# Production image, copy all the files and run Next.js
FROM base AS runner
WORKDIR /app
ENV NODE_ENV=production
# Disable telemetry during runtime if desired
# ENV NEXT_TELEMETRY_DISABLED=1
RUN addgroup --system --gid 1001 nodejs
RUN adduser --system --uid 1001 nextjs
COPY --from=builder /app/public ./public
# Leverage output traces to reduce image size
COPY --from=builder --chown=nextjs:nodejs /app/.next/standalone ./
COPY --from=builder --chown=nextjs:nodejs /app/.next/static ./.next/static
USER nextjs
EXPOSE 3000
ENV PORT=3000
ENV HOSTNAME="0.0.0.0"
# Start the Next.js server
CMD ["node", "server.js"]
Phases of the Docker Build Process
1. Base Image (base
stage)
- The
node:18-alpine
image is used for a lightweight Node.js runtime. -
libc6-compat
is installed to resolve compatibility issues with Alpine-based images.
2. Dependency Installation (deps
stage)
- Dependency installation is based on the detected lockfile (
yarn.lock
,package-lock.json
, orpnpm-lock.yaml
). - Ensures consistency by locking dependencies.
3. Application Build (builder
stage)
- Copies project files and dependencies into the container.
- Builds the Next.js application using
yarn
,npm
, orpnpm
, depending on the lockfile. - Outputs a production-ready standalone build.
4. Production Runner (runner
stage)
- Configures a production-ready environment.
- Creates a non-root user (
nextjs
) to enhance security. - Copies the necessary output (
public
,.next/standalone
, and.next/static
) from the build stage. - Exposes port 3000 and starts the Next.js server.
Key Features
1. Multi-Stage Builds
- Separates the build process into distinct stages to optimize image size and security.
- Ensures that the production image contains only the necessary runtime files.
2. Flexible Dependency Management
- Detects the appropriate package manager based on the lockfile.
- Ensures compatibility with different teams' preferences (Yarn, npm, pnpm).
3. Security Best Practices
- Runs the application as a non-root user (
nextjs
). - Uses the
alpine
base image for a minimal attack surface.
4. Reduced Image Size
- Utilizes Next.js output file tracing to include only essential files in the production image.
- Leverages
.next/standalone
for optimized deployments.
Running the Dockerized Next.js Application
Build the Image
Run the following command to build the Docker image:
docker build -t nextjs-app .
Run the Container
Start the container using:
docker run -p 3000:3000 nextjs-app
Access the application at http://localhost:3000
.
Customizations
-
Disable Telemetry: Uncomment
NEXT_TELEMETRY_DISABLED=1
to disable anonymous telemetry data collection during build and runtime. -
Environment Variables: Add environment-specific configurations using
ENV
directives or.env
files.
Conclusion
This Nextjs Dockerfile provides a comprehensive and efficient way to build and deploy Next.js applications. By leveraging multi-stage builds, output tracing, and best practices for security and performance, you can confidently deploy your Next.js projects in production environments. Adapt the Dockerfile to fit your project's unique requirements for maximum flexibility.
What's Your Reaction?
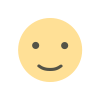
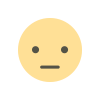
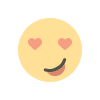
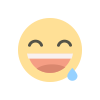
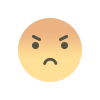
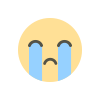
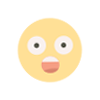