CORS Misconfigurations in Laravel: Risks and Fixes
Cross-Origin Resource Sharing (CORS) Misconfigurations in Laravel: Risks and Fixes Cross-Origin Resource Sharing (CORS) is an essential security mechanism that controls how resources on your web server are accessed by external domains. When misconfigured, it can expose your Laravel application to critical vulnerabilities, potentially leading to unauthorized data access or malicious exploits. In this post, we'll explore what CORS misconfigurations are, their implications, and how to fix them in Laravel. We'll also show how our free Website Security Scanner tool can help you identify these vulnerabilities. What is CORS and Why Does It Matter? CORS is a protocol that allows servers to define which domains can access their resources. It’s commonly implemented using HTTP headers like: Access-Control-Allow-Origin Access-Control-Allow-Methods Access-Control-Allow-Headers When properly configured, CORS prevents unauthorized external domains from making malicious requests to your web application. However, when misconfigured, it can allow unintended access and compromise your application’s security. Impacts of CORS Misconfigurations Here are the potential risks of CORS misconfigurations: Data Exposure: Sensitive user data can be accessed by malicious websites. Cross-Site Request Forgery (CSRF): Attackers can perform actions on behalf of authenticated users. Script Injection: Exploiting JavaScript APIs to perform unauthorized actions. Detecting CORS Misconfigurations in Laravel You can manually check for CORS misconfigurations using tools like curl or browser developer tools. However, a faster and more efficient way is to use our free Website Security Checker Tool. Here’s a screenshot of our tool's interface: Screenshot of the free tools webpage where you can access security assessment tools. You can visit https://free.pentesttesting.com/ to run a quick scan of your website and identify CORS issues. Common CORS Misconfigurations in Laravel Below are examples of common CORS misconfigurations and how to fix them: Example 1: Allowing All Origins (*) This is one of the most dangerous misconfigurations: return [ 'paths' => ['*'], 'allowed_methods' => ['*'], 'allowed_origins' => ['*'], 'allowed_origins_patterns' => [], 'allowed_headers' => ['*'], 'exposed_headers' => [], 'max_age' => 0, 'supports_credentials' => false, ]; This configuration allows any domain to access your resources, putting your application at risk of CSRF and other attacks. Fix: Restricting Origins and Methods Update your config/cors.php file to specify only trusted domains and methods: return [ 'paths' => ['api/*'], 'allowed_methods' => ['GET', 'POST'], 'allowed_origins' => ['https://yourdomain.com'], 'allowed_origins_patterns' => [], 'allowed_headers' => ['Content-Type', 'X-Requested-With'], 'exposed_headers' => [], 'max_age' => 3600, 'supports_credentials' => true, ]; Testing the Fix After making these changes, test your CORS configuration using our Website Vulnerability Assessment Report generated by our free tool to check Website Vulnerability. Here's an example screenshot: An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities. This report highlights vulnerabilities, including CORS misconfigurations, and suggests actionable fixes. Additional Tips for Secure CORS Configuration in Laravel Avoid Using * Wildcards: Always restrict origins, headers, and methods to specific values. Enable Credential Support Only When Necessary: Set supports_credentials to true only if your application requires it. Regularly Scan Your Application: Use automated tools like ours to detect misconfigurations and other vulnerabilities. Conclusion CORS misconfigurations can pose significant risks to your Laravel application if not addressed. By understanding the common pitfalls and applying proper configurations, you can safeguard your web application against unauthorized access and potential exploits. To make this process easier, leverage our free Website Security Checker tool. It provides a comprehensive vulnerability report, enabling you to identify and fix CORS-related issues quickly. Stay proactive and secure your Laravel applications effectively!

Cross-Origin Resource Sharing (CORS) Misconfigurations in Laravel: Risks and Fixes
Cross-Origin Resource Sharing (CORS) is an essential security mechanism that controls how resources on your web server are accessed by external domains. When misconfigured, it can expose your Laravel application to critical vulnerabilities, potentially leading to unauthorized data access or malicious exploits. In this post, we'll explore what CORS misconfigurations are, their implications, and how to fix them in Laravel.
We'll also show how our free Website Security Scanner tool can help you identify these vulnerabilities.
What is CORS and Why Does It Matter?
CORS is a protocol that allows servers to define which domains can access their resources. It’s commonly implemented using HTTP headers like:
-
Access-Control-Allow-Origin
-
Access-Control-Allow-Methods
-
Access-Control-Allow-Headers
When properly configured, CORS prevents unauthorized external domains from making malicious requests to your web application. However, when misconfigured, it can allow unintended access and compromise your application’s security.
Impacts of CORS Misconfigurations
Here are the potential risks of CORS misconfigurations:
- Data Exposure: Sensitive user data can be accessed by malicious websites.
- Cross-Site Request Forgery (CSRF): Attackers can perform actions on behalf of authenticated users.
- Script Injection: Exploiting JavaScript APIs to perform unauthorized actions.
Detecting CORS Misconfigurations in Laravel
You can manually check for CORS misconfigurations using tools like curl
or browser developer tools. However, a faster and more efficient way is to use our free Website Security Checker Tool.
Here’s a screenshot of our tool's interface:
Screenshot of the free tools webpage where you can access security assessment tools.
You can visit https://free.pentesttesting.com/ to run a quick scan of your website and identify CORS issues.
Common CORS Misconfigurations in Laravel
Below are examples of common CORS misconfigurations and how to fix them:
Example 1: Allowing All Origins (*
)
This is one of the most dangerous misconfigurations:
return [
'paths' => ['*'],
'allowed_methods' => ['*'],
'allowed_origins' => ['*'],
'allowed_origins_patterns' => [],
'allowed_headers' => ['*'],
'exposed_headers' => [],
'max_age' => 0,
'supports_credentials' => false,
];
This configuration allows any domain to access your resources, putting your application at risk of CSRF and other attacks.
Fix: Restricting Origins and Methods
Update your config/cors.php
file to specify only trusted domains and methods:
return [
'paths' => ['api/*'],
'allowed_methods' => ['GET', 'POST'],
'allowed_origins' => ['https://yourdomain.com'],
'allowed_origins_patterns' => [],
'allowed_headers' => ['Content-Type', 'X-Requested-With'],
'exposed_headers' => [],
'max_age' => 3600,
'supports_credentials' => true,
];
Testing the Fix
After making these changes, test your CORS configuration using our Website Vulnerability Assessment Report generated by our free tool to check Website Vulnerability. Here's an example screenshot:
An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
This report highlights vulnerabilities, including CORS misconfigurations, and suggests actionable fixes.
Additional Tips for Secure CORS Configuration in Laravel
-
Avoid Using
*
Wildcards: Always restrict origins, headers, and methods to specific values. -
Enable Credential Support Only When Necessary: Set
supports_credentials
totrue
only if your application requires it. - Regularly Scan Your Application: Use automated tools like ours to detect misconfigurations and other vulnerabilities.
Conclusion
CORS misconfigurations can pose significant risks to your Laravel application if not addressed. By understanding the common pitfalls and applying proper configurations, you can safeguard your web application against unauthorized access and potential exploits.
To make this process easier, leverage our free Website Security Checker tool. It provides a comprehensive vulnerability report, enabling you to identify and fix CORS-related issues quickly.
Stay proactive and secure your Laravel applications effectively!
What's Your Reaction?
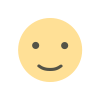
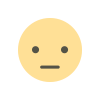
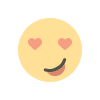
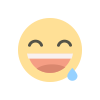
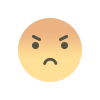
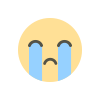
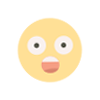