C# var vs. interface vs. concrete class in object instantiation
As we know, there are (at least) four ways to instantiate a class object in C#. But I've never quite understood why some ways are better than others. First of all, you can explicitly declare the variable as the concrete class and instantiate it as follows (this appears to be the practice of many general developers): Customer cust = new Customer(); The redundancy of this appproach has always bothered me. (Why do we need to be told twice that the the object we're creating is a Customer?) Secondly, there is the newer shorthand version of this approach, which I find much easier on my eyes: Customer cust = new(); Thirdly, there is var. var cust = new Customer(); And finally, one can code to the interface rather than the concrete class (assuming we have an interface ICustomer that Customer implements): ICustomer cust = new Customer(); This pattern is frequently used by Java programmers. The vast majority of the time I use var. But I am beginning to rethink this. It seems that there is a lot of wisdom in the fourth (Java-style) approach of coding to an interface, as it would be more dependency-injection friendly and allow one to more easily substitute out different implementations of the object. Can you think of any reason why a developer would use the explicit first approach, as opposed to the shorthand version, or var, or an interface? Or is this all just a matter of style and opinion?
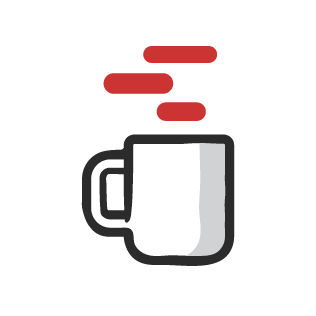
As we know, there are (at least) four ways to instantiate a class object in C#. But I've never quite understood why some ways are better than others.
First of all, you can explicitly declare the variable as the concrete class and instantiate it as follows (this appears to be the practice of many general developers):
Customer cust = new Customer();
The redundancy of this appproach has always bothered me. (Why do we need to be told twice that the the object we're creating is a Customer?)
Secondly, there is the newer shorthand version of this approach, which I find much easier on my eyes:
Customer cust = new();
Thirdly, there is var
.
var cust = new Customer();
And finally, one can code to the interface rather than the concrete class (assuming we have an interface ICustomer
that Customer
implements):
ICustomer cust = new Customer();
This pattern is frequently used by Java programmers.
The vast majority of the time I use var
. But I am beginning to rethink this. It seems that there is a lot of wisdom in the fourth (Java-style) approach of coding to an interface, as it would be more dependency-injection friendly and allow one to more easily substitute out different implementations of the object.
Can you think of any reason why a developer would use the explicit first approach, as opposed to the shorthand version, or var
, or an interface? Or is this all just a matter of style and opinion?