Building a Random Quotes Generator: A Step-by-Step Guide with Code
Creating a Random Quotes Generator App is a great way to learn coding by building something practical. This guide explains the steps I followed to create the app, including detailed code snippets to help beginners understand the process at the code level. Project Overview The app fetches random quotes using a public API, displays them on the screen, and allows users to copy or share the quotes. We’ll break down the steps to build this app and discuss the logic behind the code. Step 1: Setting Up the HTML Structure The first step is creating the HTML layout for the app. Here’s the basic structure: Random Quotes Generator Random Quotes Generator "Randomness is not the absence of order, but the presence of possibilities." Quote will appear here Author unknown New Quote Credit: Quotes API Built with ♥ from Dev This code defines the structure of the app, including elements to display the quote, buttons to fetch new quotes, and icons for copy and share functionality. Step 2: Handling CORS Issues with a Proxy Server To fetch quotes from an external API, you need to handle CORS (Cross-Origin Resource Sharing). We achieve this using a simple Express.js proxy server. proxy.js: const express = require("express"); const fetch = require("node-fetch"); const cors = require("cors"); const app = express(); // Enable CORS app.use(cors()); // Define a route for proxying API requests app.get("/api/quote", async (req, res) => { try { const response = await fetch("https://qapi.vercel.app/api/random"); const data = await response.json(); res.json(data); } catch (error) { res.status(500).json({ error: "Failed to fetch data from API" }); } }); const PORT = 4000; app.listen(PORT, () => console.log(`Proxy server running on http://localhost:${PORT}`)); This acts as a local proxy to fetch quotes from the API and bypass CORS restrictions. Step 3: Fetching Quotes with JavaScript The app fetches a new quote when the "New Quote" button is clicked. Here’s how the logic works: index.js: const quote = document.querySelector(".quote"); const author = document.querySelector(".author"); async function generateQuote() { const url = 'http://localhost:4000/api/quote'; try { const response = await fetch(url); if (!response.ok) { throw new Error(`HTTP error! Status: ${response.status}`); } const result = await response.json(); quote.textContent = result.quote || "No quote available."; author.textContent = result.author || "Author Unknown"; } catch (error) { console.error("Error fetching quote:", error); quote.textContent = "Failed to fetch quote. Try again later."; } } This script fetches data from the proxy server and updates the app’s UI with the fetched quote and author. Step 4: Adding the Copy Feature To enable users to copy quotes, we use the Clipboard API. copyQuote.js: async function copyQuote() { try { let quote = document.querySelector(".quote").textContent; let author = document.querySelector(".author").textContent; await navigator.clipboard.writeText(`${quote} - ${author}`); alert("Content copied to clipboard"); } catch (error) { console.error('Failed to copy:', error); } } When the user clicks the copy icon, the quote and author are copied to the system clipboard. Step 5: Adding the Share Feature The Navigator API allows users to share quotes on WhatsApp. shareQuote.js: async function shareQuote() { let quote = document.querySelector(".quote").textContent; let author = document.querySelector(".author").textContent; const quoteContent = `${quote} - ${author}`; const message = encodeURIComponent(quoteContent); if (window.screen.width

Creating a Random Quotes Generator App is a great way to learn coding by building something practical. This guide explains the steps I followed to create the app, including detailed code snippets to help beginners understand the process at the code level.
Project Overview
The app fetches random quotes using a public API, displays them on the screen, and allows users to copy or share the quotes. We’ll break down the steps to build this app and discuss the logic behind the code.
Step 1: Setting Up the HTML Structure
The first step is creating the HTML layout for the app. Here’s the basic structure:
lang="en">
charset="UTF-8">
name="viewport" content="width=device-width, initial-scale=1.0">
Random Quotes Generator
rel="stylesheet" href="styles.css">
class="quote-generator">
class="title">Random Quotes Generator
class="subheading">"Randomness is not the absence of order, but the presence of possibilities."
class="quotebox">
class="quote">Quote will appear here
class="author">Author unknown
class="copyquote" onclick="copyQuote()">
src="images/copy.png" class="icon-style" alt="Copy Icon">
class="sharequote" onclick="shareQuote()">
src="images/share.png" class="icon-style" alt="Share Icon">
class="footer">
class="footer-left">
class="credits" href="https://github.com/theriturajps/Quotes-API" target="_blank" rel="noopener">Credit: Quotes API
class="footer-right">
href="https://github.com/theriturajps/Quotes-API" class="menu" target="_blank" rel="noopener">
src="images/dev_github.png" class="icon-style" alt="GitHub Icon">
href="https://github.com/theriturajps/Quotes-API" class="menu" target="_blank" rel="noopener">
src="images/dev_x_twitter.png" class="icon-style" alt="Twitter Icon">
href="https://github.com/theriturajps/Quotes-API" class="menu" target="_blank" rel="noopener">
src="images/dev_linkedin.png" class="icon-style" alt="LinkedIn Icon">
Built with ♥ from Dev
This code defines the structure of the app, including elements to display the quote, buttons to fetch new quotes, and icons for copy and share functionality.
Step 2: Handling CORS Issues with a Proxy Server
To fetch quotes from an external API, you need to handle CORS (Cross-Origin Resource Sharing). We achieve this using a simple Express.js proxy server.
proxy.js:
const express = require("express");
const fetch = require("node-fetch");
const cors = require("cors");
const app = express();
// Enable CORS
app.use(cors());
// Define a route for proxying API requests
app.get("/api/quote", async (req, res) => {
try {
const response = await fetch("https://qapi.vercel.app/api/random");
const data = await response.json();
res.json(data);
} catch (error) {
res.status(500).json({ error: "Failed to fetch data from API" });
}
});
const PORT = 4000;
app.listen(PORT, () => console.log(`Proxy server running on http://localhost:${PORT}`));
This acts as a local proxy to fetch quotes from the API and bypass CORS restrictions.
Step 3: Fetching Quotes with JavaScript
The app fetches a new quote when the "New Quote" button is clicked. Here’s how the logic works:
index.js:
const quote = document.querySelector(".quote");
const author = document.querySelector(".author");
async function generateQuote() {
const url = 'http://localhost:4000/api/quote';
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const result = await response.json();
quote.textContent = result.quote || "No quote available.";
author.textContent = result.author || "Author Unknown";
} catch (error) {
console.error("Error fetching quote:", error);
quote.textContent = "Failed to fetch quote. Try again later.";
}
}
This script fetches data from the proxy server and updates the app’s UI with the fetched quote and author.
Step 4: Adding the Copy Feature
To enable users to copy quotes, we use the Clipboard API.
copyQuote.js:
async function copyQuote() {
try {
let quote = document.querySelector(".quote").textContent;
let author = document.querySelector(".author").textContent;
await navigator.clipboard.writeText(`${quote} - ${author}`);
alert("Content copied to clipboard");
} catch (error) {
console.error('Failed to copy:', error);
}
}
When the user clicks the copy icon, the quote and author are copied to the system clipboard.
Step 5: Adding the Share Feature
The Navigator API allows users to share quotes on WhatsApp.
shareQuote.js:
async function shareQuote() {
let quote = document.querySelector(".quote").textContent;
let author = document.querySelector(".author").textContent;
const quoteContent = `${quote} - ${author}`;
const message = encodeURIComponent(quoteContent);
if (window.screen.width < 768 || navigator.userAgent.match(/Android|iOS/)) {
window.open(`whatsapp://send?text=${message}`, '_blank');
} else {
window.open(`https://web.whatsapp.com/send?text=${message}`, '_blank');
}
}
This script adapts sharing functionality for both mobile and desktop devices.
Step 6: Styling the App
Using CSS, I styled the app to make it visually appealing and responsive. Key aspects include centering elements and designing for various screen sizes.
html, body {
background: rgb(171,129,205);
background: linear-gradient(0deg, rgba(171,129,205,1) 0%, rgba(34,42,104,1) 100%);
margin: 0;
padding: 0;
height: 100%;
}
main {
flex: 1; /* Fills available space */
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
.title, .subheading {
align-items: center;
text-align: center;
color: #fafffd
}
.subheading {
align-items: center;
text-align: center;
color: #fa824c
}
p {
text-align: center;
}
.quote-generator, .quote-box, .footer {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
margin: 100 auto;
margin-top: 50px;
}
.quotebox {
width: 300px auto;
height: 200px auto;
margin-top: 100px;
padding: 10px;
border: 2px solid #9fd356;
overflow: auto;
border-radius: 20px;
}
.quote {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
margin: 100 auto;
color: #fafffd;
}
.quote-button {
padding: 15px;
margin: 15px;
border-radius: 5px;
background-color: #e2adf2;
color: black;
font-size: larger;
}
.copyquote, .sharequote {
display: flex;
}
.author {
color: #9fd356;;
}
.footer {
display: flex;
flex-direction: column;
align-items: center;
justify-content: end;
height: 200px;
margin: 200px;
}
.footer-left {
font-size: 20px;
float: center;
}
.footer-right {
margin-top:20px;
float:center;
}
a.menu:hover {
border-radius: 2px;
font-size: 20px;
}
.icon-style {
height:60px;
margin-left:20px;
margin-top:5px;
}
.icon-style:hover {
background-color: #ab81cd;
padding:5px;
border-radius: 15px;
transition: 0.5s;
transition-duration: 0.25s;
color: azure;
}
Step 7: Launching the App
The app can be built from the example code. Alternately, the subsequent steps may be followed to attempt the application.
To launch and use the app, follow these steps:
- Clone the Repository:
git clone https://github.com/yourusername/random-quotes-generator.git
cd random-quotes-generator
- Install Dependencies: Make sure you have Node.js installed on your system. Then run:
npm install
- Start the Proxy Server: Run the following command to start the proxy server:
node proxy.js
The proxy server will run on http://localhost:4000
.
-
Open the App:
Open the
index.html
file in your browser to interact with the app. Use the "New Quote" button to fetch quotes, copy them using the copy icon, or share them via WhatsApp using the share icon.
Project Structure:
index.html
: Main UI for the app.
proxy.js
: Proxy server to handle CORS issues.
copyQuote.js
: Handles copying quotes to the clipboard.
shareQuote.js
: Handles sharing quotes via WhatsApp.
index.js
: Core functionality for fetching and displaying quotes.
API Credit:
Quotes provided by Quotes API.
Conclusion
This guide demonstrates how to build a Random Quotes Generator app, covering essential steps like fetching API data, handling CORS, and implementing copy and share features. It can help:
- Understand API integration and CORS issues.
- Explore browser APIs like Clipboard and Navigator.
- Practice JavaScript fundamentals.
Happy coding! I am a learner and would love to hear feedback from the community and experienced developers. Your suggestions are valuable to me, and I am eager to learn and improve. You are welcome to reach out!
What's Your Reaction?
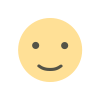
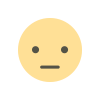
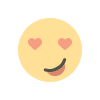
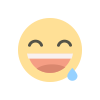
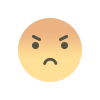
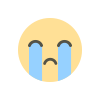
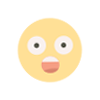